Python for Loop
A loop constitutes a foundational programming concept frequently employed in software development. It entails a sequence of instructions that is reiterated until a specific condition is met. Within a for loop, there are two components: a header defining the conditions for iteration and a body executed with each iteration. Typically, the header includes a designated loop counter or variable, enabling the body to discern the ongoing iteration.
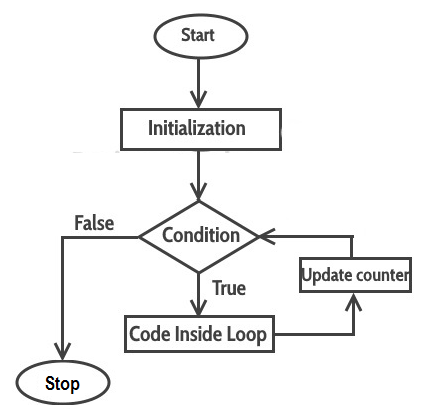
Using for loop in Python List
In this scenario, the variable "directions" encompasses a sequence comprising a list of directions. Upon executing the "for" loop, the initial item (North) is assigned to the variable "pole." Subsequently, the print statement is executed, and this sequence persists until the iteration covers the entire list.
Using for loop in Python Tuple
Using for loop in Python Dictionary
Using for loop in Python String
Python for loop range() function
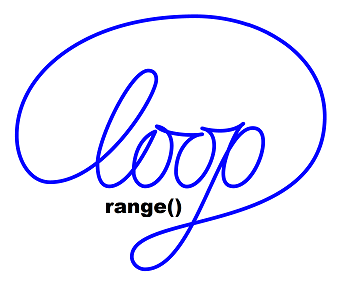
The range function within a "for" loop serves as a potent tool for generating sequences of integers. It can accept one, two, or three arguments, generating a list of integers starting from a lower bound (default is zero) and extending up to (but not inclusive of) an upper limit, with optional intervals (default is one). Noteworthy for Python 3 users is the absence of separate range and xrange() functions; in Python 3, only range exists, aligning with the design of Python 2's xrange.
- range(stop)
- range(start,stop)
- range(start,stop,step)
It is important to note that all parameters must be integers and can be positive or negative .
Python range() function with one parameters
Syntax- stop: Generate numbers up to, but not including this number.
It can be thought of working like this:
So you can see that i gets the value 0, 1, 2, 3, 4 at the same time, but rather sequentially.
Python range() function with two parameters
Syntax- start: Starting number of the sequence.
- stop: Generate numbers up to, but not including this number.
The range(start,stop) generates a sequence with numbers start, start + 1, ..., stop - 1. The last number is not included.
Python range() function with three parameters
Syntax- start: Starting number of the sequence.
- stop: Generate numbers up to, but not including this number.
- step: Difference between each number in the sequence.
Here the start value is 0 and end values is 10 and step is 3. This means that the loop start from 0 and end at 10 and the increment value is 3.
Python Range() function can define an empty sequence, like range(-10) or range(10, 4). In this case the for-block won't be executed:
exampleThe above code won't be executed.
Also, you can use Python range() for repeat some action several times:
exampleDecrementing for loops
If you want a decrementing for loops , you need to give the range a -1 step
exampleAccessing the index in 'for' loops in Python
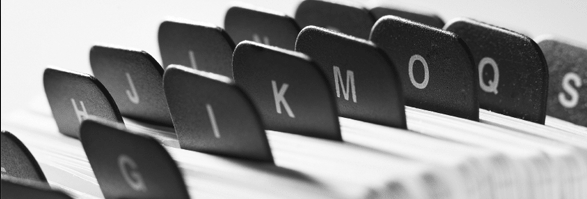
Python's native "enumerate" function provides a convenient mechanism for iterating through a list while simultaneously retrieving both the index and the corresponding value of each item in the list. This function simplifies code presentation by abstracting the index management, encapsulating the iterable into a new iterable that yields two-item tuples containing the index and item. This enhances code readability and streamlines the process of handling iterable objects.
exampleNote: the start=1 option to enumerate here is optional. If we didn't specify this, we'd start counting at 0 by default.
Python enumerate function perform an iterable where each element is a tuple that contains the index of the item and the original item value.
So, this function is meant for:
- Accessing each item in a list (or another iterable).
- Also getting the index of each item accessed.
Iterate over two lists simultaneously
In the following Python program we're looping over two lists at the same time using indexes to look up corresponding elements.
exampleUsing Python zip() in for loop
The Python zip() function takes multiple lists and returns an iterable that provides a tuple of the corresponding elements of each list as we loop over it.
Nested for loop in Python
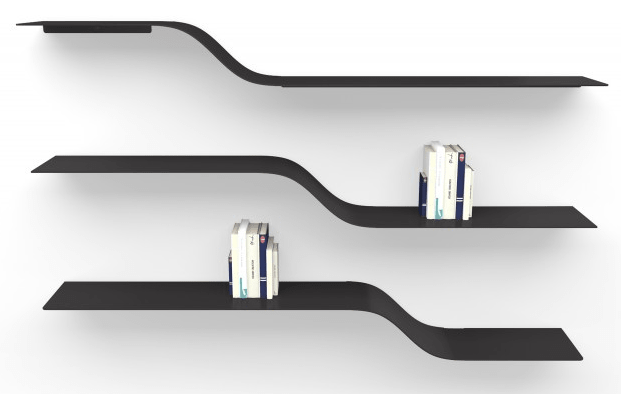
Syntax
The initial focus is on the outer loop, initiating its first iteration. Subsequently, this triggers the nested loop, which proceeds to run its course. Upon completion of the nested loop, the program revisits the outer loop to finalize its second iteration, consequently reactivating the nested loop. This pattern persists, with the nested loop being executed fully each time, until the entire sequence concludes or an intervening statement like "break" disrupts the sequence. Within the inner loop's print() function, the second parameter "end=' '" appends a space instead of the default newline, causing the numbers to appear in a single row.
Using else statement in python for loop
Unlike other popular programming languages, Python also allows developers to use the else condition with for loops.
exampleIn the above example, there is list with integer number. While entering the for loop each number is checked with greater than 5 or not. Here you can see the else executes only if break is NEVER reached and loop terminated after all iterations.
Conclusion
The Python "for" loop facilitates sequential iteration through a sequence, such as a list or range, enabling access to both index and value in each iteration using the "enumerate" function. It offers a concise mechanism for performing repetitive tasks and handling iterable objects with enhanced readability and ease.