with statement in Python
In Python, the "with" statement streamlines exception handling through the use of context managers, simplifying common preparation and cleanup tasks. This approach encapsulates try..except..finally patterns for reuse, reducing code required for diverse exception handling. Within a block, resources are established by the "with" statement, allowing you to operate on them. Upon block exit, resources are systematically released, regardless of whether the exit is normal or due to an exception, ensuring clean resource management.
SyntaxThe "with" statement employs an __enter() and an __exit() function, invoked at the statement's inception and conclusion. The object's enter() executes before the with-block and can initialize setup code, potentially returning a value bound to a variable. Once the with-block concludes, the object's exit() is invoked, even in case of exceptions, enabling execution of cleanup tasks. This mechanism shares similarities with the "using statement" in .NET languages, simplifying resource management.
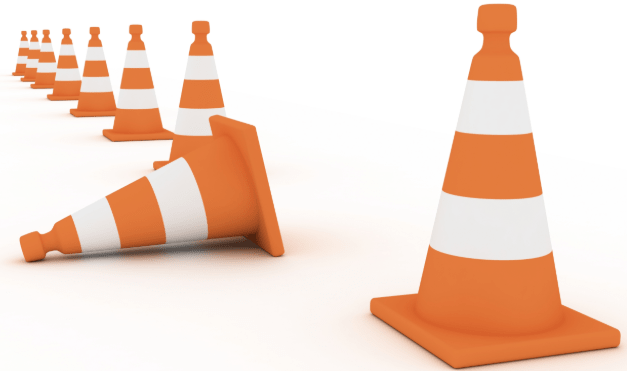
With Statement Usage
In many instances, the Python "with" keyword finds relevance particularly in scenarios involving unmanaged resources, such as file streams. It offers a form of syntactic enhancement akin to that provided by try...finally blocks. To illustrate, consider the subsequent example employing the try, except, and finally statements to handle the process of opening and reading a file named "myFile.txt."
exampleThe following example using the Python "with statement" .
example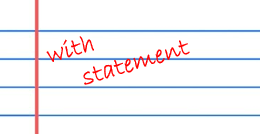
In the above example, the "with" statement ensures automatic closure of the file upon completion of the nested code block. The notable benefit of employing a "with" statement lies in its guarantee of file closure, irrespective of how the nested block concludes. This mechanism remains consistent even in situations where an exception is encountered before the block's conclusion, as the file is closed prior to being caught by an outer exception handler. Additionally, if the nested block incorporates a "return," "continue," or "break" statement, the "with" statement ensures file closure under those circumstances as well.
with statement in Threading
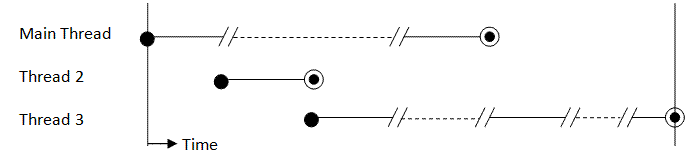
example
Numerous resources within the Python library adhere to the protocol mandated by the "with" statement, rendering them readily compatible with its usage. Employ the "with" statement whenever your application acquires resources demanding explicit release, such as files, network connections, locks, and similar entities. This approach ensures efficient and reliable resource management.
Conclusion
The "with" statement in Python streamlines context management by automatically handling resource acquisition and release, such as files or connections. It ensures proper cleanup and enhances code readability, making it a preferred choice for managing resources that require explicit relinquishing.