Running a .BAT file
What is a .Bat file?
A batch (.bat) file is a script containing a sequence of DOS commands, carefully crafted to streamline and automate routine tasks frequently executed on a computer system. These files, denoted by the .bat extension, serve as powerful tools, facilitating a seamless amalgamation of multiple commands into a single script.
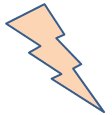
By using the potential of batch files, users can optimize their workflow and boost productivity through the automation of specific actions, ensuring that essential tasks are accomplished with a mere click, sparing valuable time and effort in the process. The ease of execution and the ability to encapsulate complex procedures within a single file make batch files an indispensable asset in the scope of system administration and task management, allowing users to focus on more critical aspects of their work with heightened efficiency and precision.
How to create a .Bat file?
To craft a straightforward and efficient batch file, a single command that you wish to execute is all that's required. This command can be carefully entered into a plain text file, and then, to ensure its proper functionality, it is diligently saved with the .BAT extension, such as "testbat.bat." By following this straightforward procedure, you can promptly create a potent and versatile batch file that streamlines your desired command's execution, maintaining seamless automation and enhancing operational efficacy. Utilizing batch files in this manner can expedite routine tasks, improve productivity, and empower users to optimize their workflows with ease and precision, rendering them valuable tools for system administrators and users seeking to streamline repetitive actions.
The following .bat file create a folder "d:\MyDir" in your D drive. The content of the file as follows :
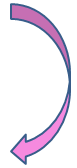
To initiate the process, access the Notepad application and carefully copy and paste the provided content into the text editor. Next, diligently save the file with the appellation "testbat.bat" to ensure proper identification as a batch file. For prompt execution, a simple double-click action on the aforementioned file (testbat.bat) will activate its functionality. Upon execution, a novel directory bearing the name "MyDir" will be generated within your D drive, resulting in a seamless and efficient creation of the designated directory.
In some situations you have to run .bat files from your C# or VB.Net applications. The following programs shows how to run a .bat file from C# or VB.Net.
Source Code | C#
Source Code | Vb.Net
Start and Kill Processes
Using the Process component, you can obtain a list of the processes that are running, or you can start a new process in the system. The System.Diagnostics namespace provides classes that allow you to interact with system processes, event logs, and performance counters. More about..... Start and Kill Processes
How get Running Process List
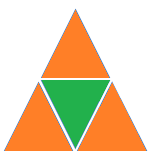
The Process class provides functionality to monitor system processes across the network, and to start and stop local system processes. You can retrieve detailed knowledge of process threads and modules both through the Process class itself. More about...... Running Process List
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#