Convert byte array to hex string
Byte array
A byte encompasses 8 bits, and a byte array, as the name suggests, constitutes an array of bytes. This byte array proves indispensable for storing binary data collections, encompassing diverse sources like data files, image files, compressed files, downloaded server responses, and an array of other file types. However, it is essential to consider employing streaming data types when handling substantial volumes of binary data, particularly if the programming language supports such a feature. Nonetheless, byte arrays present an optimal means of representation for this data, as they efficiently capture low-level representations, offering valuable flexibility in method optimization. By utilizing byte arrays, developers can utilize the power of low-level data handling, enhancing the performance of certain operations and ensuring the efficient storage and manipulation of binary information.
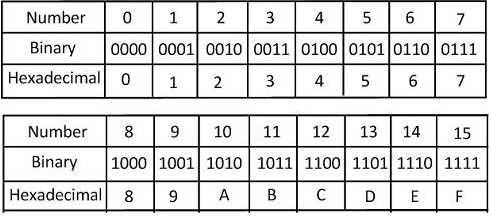
Hexadecimal string
Characters are represented as ASCII and they are 1 byte long, a byte could be represented with 2 digits in hexadecimal format for example 255 is 0xFF.
Convert a byte array to a hexadecimal string
If you want it without the dashes, just remove them:
Convert a String Hex to byte array
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to Catch multiple exceptions with C#