Byte array to string in C#
In C#, you can convert a byte array to a string using different encodings. When you convert a byte array to a string, you are essentially decoding the bytes back into their original character representation. Let's explore two common methods to achieve this:
Using Encoding.GetString() Method
You can use the Encoding.GetString() method to convert a byte array to a string. This method takes the byte array as input and returns the corresponding string representation.
In this example, the byte array contains the ASCII values of the characters "Hello." The GetString() method with the Encoding.UTF8 argument decodes the bytes using the UTF-8 encoding and returns the string representation.
Using Convert.ToBase64String()
If you have a byte array that represents binary data (e.g., images, files), you can use Convert.ToBase64String() to convert the byte array to a Base64 string.
In this example, the byte array is converted to its Base64 string representation. Base64 encoding is commonly used to represent binary data in a textual format.
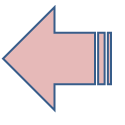
Keep in mind that when converting a byte array to a string, the correct encoding must be used to interpret the bytes correctly. In the first method, Encoding.GetString() uses the specified encoding to convert the bytes to characters. In the second method, Convert.ToBase64String() converts the bytes to a textual representation using Base64 encoding.
Conclusion
When decoding a byte array back to a string, make sure to use the same encoding that was used during the conversion from the string to the byte array. This ensures that the characters are correctly interpreted, and you get the original string back.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#