Custom Exception in C#
In C#, exception handling provides a mechanism to deal with errors or exceptional situations that can occur during the execution of a program. C# allows developers to create their own custom exceptions to handle specific error scenarios that are not covered by the built-in exception classes.
Creating Custom Exceptions
To create a custom exception in C#, you need to define a new class that derives from the System.Exception class. By doing this, your custom exception class will inherit all the properties and methods of the base Exception class, making it easy to customize the behavior as per your requirements.
Following is an example of creating a custom exception named InvalidInputException:
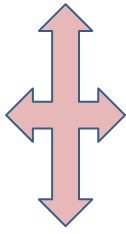
In the above example, the InvalidInputException class is derived from Exception. It includes three constructors: a default constructor, one that takes a custom error message, and one that takes both a custom error message and an inner exception (used to represent the original exception that caused this custom exception).
Using Custom Exceptions
Once you have defined your custom exception, you can use it in your code to handle specific error scenarios. For example, let's say you have a method that calculates the area of a rectangle, and you want to throw a custom exception if the input parameters (length and width) are negative.
In the above code, when the CalculateArea method is called with negative values for length or width, it throws an InvalidInputException with the specified error message.
Handling Custom Exceptions
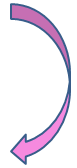
To handle custom exceptions or any exceptions in C#, you use try-catch blocks. When an exception is thrown, the runtime searches for a catch block that can handle that exception type. If it finds a matching catch block, the code inside the catch block is executed.
In this example, the Main method calls the CalculateArea method with negative values. Since the method throws an InvalidInputException, the catch block for InvalidInputException will handle it, and the custom error message will be displayed. If there were other types of exceptions, the generic Exception catch block would handle them.
Conclusion
Custom exceptions allow you to create more meaningful error messages and provide better context about what went wrong in your program. They make debugging and error resolution more efficient, especially when working on large-scale projects.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#