Iterate over a Dictionary
In C#, a Dictionary<TKey, TValue> is a collection that stores key-value pairs, where each key is unique. When iterating over a dictionary, there are several methods available, each with its advantages and use cases. Here are the best ways to iterate over a dictionary in C#:
In C# 3.0 and later versions, you can use the var keyword to infer the type of the loop variable automatically. This approach simplifies the code and makes it more concise.
Choosing the best method for iterating over a dictionary depends on your specific use case. If you need to work with both keys and values, using the foreach loop with KeyValuePair<TKey, TValue> is generally the most suitable and straightforward approach. However, if you only need to process keys or values, you can use the Keys or Values properties. Additionally, if you require more complex filtering or projection, the LINQ methods provide powerful and flexible options for working with dictionaries.
Using foreach loop with KeyValuePair<TKey, TValue>
The most common and straightforward way to iterate over a dictionary is by using a foreach loop. The loop iterates through each key-value pair in the dictionary, represented by the KeyValuePair<TKey, TValue> structure.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Dictionary<string, int> myDictionary = new Dictionary<string, int>
{
{ "apple", 5 },
{ "banana", 3 },
{ "orange", 8 }
};
foreach (KeyValuePair<string, int> kvp in myDictionary)
{
Console.WriteLine($"Key: {kvp.Key}, Value: {kvp.Value}");
}
}
}
//Output:
Key: apple, Value: 5
Key: banana, Value: 3
Key: orange, Value: 8
Using foreach loop with var
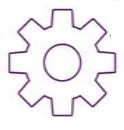
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Dictionary<string, int> myDictionary = new Dictionary<string, int>
{
{ "apple", 5 },
{ "banana", 3 },
{ "orange", 8 }
};
foreach (var kvp in myDictionary)
{
Console.WriteLine($"Key: {kvp.Key}, Value: {kvp.Value}");
}
}
}
//Output:
Key: apple, Value: 5
Key: banana, Value: 3
Key: orange, Value: 8
Using foreach loop on keys or values
If you only need to iterate over the keys or values of the dictionary, you can use the Keys or Values properties of the dictionary.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Dictionary<string, int> myDictionary = new Dictionary<string, int>
{
{ "apple", 5 },
{ "banana", 3 },
{ "orange", 8 }
};
foreach (string key in myDictionary.Keys)
{
Console.WriteLine($"Key: {key}, Value: {myDictionary[key]}");
}
}
}
//Output:
Key: apple, Value: 5
Key: banana, Value: 3
Key: orange, Value: 8
Using LINQ methods (e.g., foreach, Select, Where, etc.)
The LINQ (Language-Integrated Query) library provides a set of extension methods that can be used to query and manipulate collections, including dictionaries. You can use LINQ methods like Where, Select, and ForEach to filter or project dictionary elements as needed.
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main()
{
Dictionary<string, int> myDictionary = new Dictionary<string, int>
{
{ "apple", 5 },
{ "banana", 3 },
{ "orange", 8 }
};
// Filtering and printing only key-value pairs where the value is greater than 4
foreach (var kvp in myDictionary.Where(kvp => kvp.Value > 4))
{
Console.WriteLine($"Key: {kvp.Key}, Value: {kvp.Value}");
}
}
}
//Output:
Key: apple, Value: 5
Key: orange, Value: 8
Conclusion
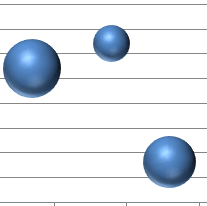
NEXT..... String to byte Array