Convert enum to List
An enumeration, often referred to as an enum type, is a data type that holds an internal list of enumerators. Enumerations offer a convenient approach to group together a set of logically related constants. When you encounter a scenario where you have multiple constants that are conceptually connected to each other, using an enum becomes the most suitable and organized method to consolidate these constants within a single entity.
Enum to List
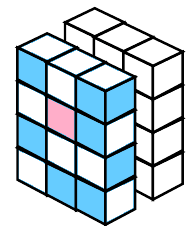
By encapsulating related constants in an enum, you create a distinct and self-contained set of values that represent different symbolic names for specific integral values. This promotes code readability, maintainability, and enhances the clarity of the codebase. It also helps prevent errors and ensures consistency when dealing with related constants throughout the program.
C# Syntax:
VB.Net Syntax:
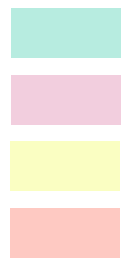
In many scenarios, it is considered good practice to define an enum directly within a namespace. By doing so, all classes within that namespace gain equal accessibility and convenience to utilize the enum without any additional qualifications. This approach promotes code modularity and ensures that related constants are easily accessible throughout the namespace, enhancing code clarity and maintainability.
However, there might be situations where you need to convert enum values into a List data structure for programming convenience. For example, when you want to perform bulk operations, iterate through enum values, or use LINQ queries, converting the enum to a List simplifies these tasks. The following program shows how to convert an enum to List in C# as well as VB.Net
C# Enum to List
VB.Net Enum to List
Conclusion
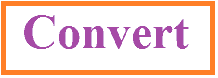
Enums are a powerful tool in programming that facilitates the grouping of logically related constants, offering a more structured and intuitive approach to manage and reference these constants within the code. Their usage contributes to code quality, organization, and overall robustness of software systems.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#