Best way to break long strings
Verbatim String Literal
A verbatim string literal in C# is denoted by an "@" character preceding a double-quote character. It allows the inclusion of escape characters and new lines without the need for escape sequences. A verbatim string literal begins with @" and concludes with a closing double-quote character. This unique string representation permits the inclusion of zero or more characters, including line breaks, tabs, and backslashes, exactly as they are written, without the need for escaping special characters. Verbatim string literals are particularly useful for defining paths, regular expressions, XML content, and any scenario where preserving the exact format is essential for readability and code maintenance.
Multiline String Literals
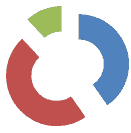
Have you encountered situations where the need to break a lengthy string into multiple lines arises? In the field of programming, this challenge is skillfully addressed through the use of Multiline String Literals, an elegant and efficient approach. By simply appending the "@" symbol before the literal, developers gain the ability to effortlessly span a string across various lines without the burden of employing escape characters or concatenation. Embracing Multiline String Literals significantly enhances the code's readability and maintainability, facilitating clear and organized presentation of textual content. Moreover, this feature effectively disables the escape mechanism, further streamlining the process and ensuring an unadulterated representation of the string. In sum, the utilization of Multiline String Literals proves invaluable for developers seeking a seamless and elegant solution to managing extensive textual data.
The following example demonstrates how to construct a multiline string literal.
Method 1: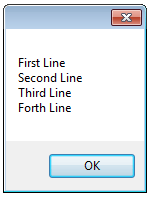
VB.Net
Method 1:In VB.Net you can use XML Literals to achieve a similar effect:
If you have any special characters included in the string , you should use a CDATA block.
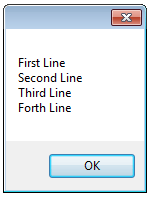
Escape double quotes in string
A verbatim string literal in C# is characterized by the presence of the "@" symbol, immediately followed by a double-quote character, followed by zero or more characters, and ultimately concluded with another closing double-quote character. This unique notation enables the representation of strings across multiple lines, making it easier to maintain readability and organization in the code. Additionally, a significant advantage of verbatim string literals lies in their capability to disable the escape mechanism. This means that any escape sequences present within the string, such as "", are treated as ordinary characters rather than special control characters. The combination of multiline support and escape sequence deactivation makes verbatim string literals an invaluable tool for programmers seeking elegant and hassle-free string representation in their C# code.
C#In VB.Net you put the quotes within another double quote.