Connect C# to MySQL
Connect C# to MySQL
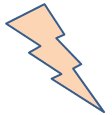
To establish a connection between a C# application and a MySQL database, MySQL offers a comprehensive set of classes within the MySQL Connector/Net. All intercommunication between the C# application and the MySQL server is channeled through a specialized MySqlConnection Object. As a prerequisite, prior to enabling communication with the server, the application is required to instantiate, configure, and successfully open a MySqlConnection object.
Download MySQL Connector/Net
You can download MySQL Connector/Net from Mysql developer website free of cost. Click the following link.... Download MySQL Connector/Net
Add Reference
Before commencing the process of connecting your application to MySql, it is imperative to include the required MySQL Reference in your project. To accomplish this, simply right-click on your project name, then proceed to select "Add Reference," followed by choosing "MySql.Data" from the list of available references. This action ensures the necessary MySQL libraries are accessible for the successful integration of the database within your application.
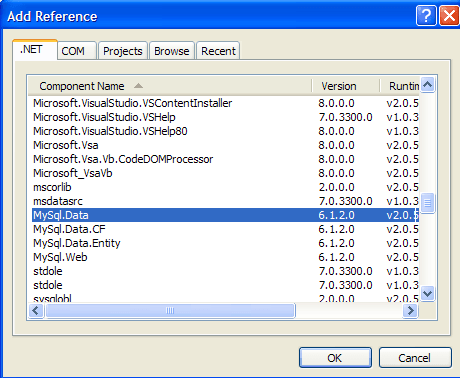
Next, you need to add MySql Library in your C# project.
C# MySQL connection string
The following C# Program is used to create a MySqlConnection object, assign the connection string, and open the connection.
Multiple servers
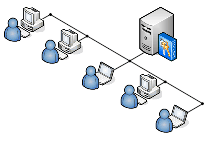
The following connection string will needed when connect to a server in a replicated server configuration without concern on which server to use.
Specifying TCP port
The defaqult MySql port is 3306 and if you use Unix socket the value will ignored.
Microsoft products
MySQL Connector/Net does not support Express versions of Microsoft products, including Microsoft Visual Web Developer (now known as Visual Studio Community). The MySQL Connector/Net is designed to work with the full versions of Microsoft products, such as Visual Studio Professional and Visual Studio Enterprise.
If you are using the Express version of Visual Studio (e.g., Visual Studio Community Edition), you can still work with MySQL databases using alternative methods. One common approach is to use Entity Framework Core, which is a lightweight, open-source ORM (Object-Relational Mapping) framework that supports various database providers, including MySQL.
To work with MySQL in a Visual Studio Community Edition or other Express versions, you can follow these steps:
- Install MySQL Connector/Net: Even though the Connector/Net does not officially support Express versions, you can still download and install the Connector/Net separately from the MySQL website. This will provide the necessary ADO.NET driver for connecting to MySQL databases.
- Use Entity Framework Core: Entity Framework Core is a cross-platform ORM that allows you to work with databases in a more abstract and code-focused manner. You can install the MySQL provider for Entity Framework Core using NuGet packages and then use EF Core for database operations.
- Configure Entity Framework Core: After installing the MySQL provider for EF Core, you need to configure your application to use MySQL as the database provider in the DbContext.
- Connect to MySQL: With the configuration in place, you can connect to your MySQL database and perform database operations using the DbContext and LINQ queries.
For the most current and up-to-date information on MySQL Connector/Net and its compatibility with different versions of Microsoft products, including Visual Studio Community Edition, it is recommended to refer to the official MySQL documentation and relevant Microsoft resources. Additionally, consider checking for any updates or changes in compatibility with future releases of both MySQL Connector/Net and Microsoft products.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#