Remove "\r\n" from a string C#
In C#, there are several ways to remove newline characters from a string. Newline characters can include the carriage return (\r), line feed (\n), or a combination of both (\r\n). Here are some common methods to achieve this:
Using String.Replace()
You can use the String.Replace() method to replace newline characters with an empty string:
In this example, we first remove the line feed (\n) and then the carriage return (\r) by replacing them with an empty string.
Using Regex.Replace()
If you have complex newline patterns or multiple variations of newlines, you can use regular expressions to remove them:
In this example, the regular expression pattern \r\n?\n matches both \r\n and \n newlines and replaces them with an empty string.
Using Environment.NewLine
If you want to remove all types of newline characters based on the platform's newline representation, you can use Environment.NewLine:
The Environment.NewLine property represents the newline characters used in the current environment (e.g., \r\n on Windows, \n on Unix).
Using LINQ and Char.IsControl()
If you only want to remove control characters (which include newlines), you can use LINQ with the Char.IsControl() method:
This method removes any control characters, including newlines, by creating a new string from the filtered characters using LINQ.
Conclusion
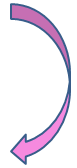
Regardless of the method you choose, remember that strings in C# are immutable, so all these approaches will create a new string without the newline characters rather than modifying the original string. Choose the method that suits your specific requirements and consider using StringBuilder if you need to perform multiple string manipulations for better performance.