C# Root Class
In .NET, the "Root class" typically refers to the most fundamental and base class in the hierarchy of all classes within the .NET framework. This class is the root of the inheritance tree, from which all other classes are derived. The root class serves as a foundation upon which all other classes are built, and it provides essential functionalities and characteristics inherited by all other classes in the .NET ecosystem.
In C#, the root class is represented by the System.Object class. Every class in C# automatically derives from System.Object unless it explicitly specifies a different base class. The System.Object class is defined in the mscorlib.dll assembly and resides within the System namespace.
Key aspects of the System.Object
Methods and Properties
The System.Object class defines several critical methods and properties that are inherited by all other classes. Some of the commonly used methods include:
- ToString(): Returns a string representation of the object.
- Equals(Object obj): Determines whether the current object is equal to the specified object.
- GetHashCode(): Returns a hash code for the object, used in hashing and collection classes.
- GetType(): Retrieves the Type of the current instance.
Boxing and Unboxing
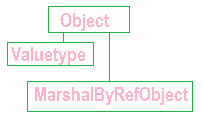
Another crucial concept related to the root class is boxing and unboxing. When a value type (e.g., int, double, etc.) is assigned to a variable of type System.Object, it undergoes "boxing," which means it gets converted to an object. Conversely, when the value is extracted from the object and assigned to a value type variable, it undergoes "unboxing."
Implicit Base Class
If a class is defined without explicitly specifying a base class, it implicitly inherits from System.Object.
Object Reference
Every object in .NET, regardless of its class, can be treated as an instance of the System.Object class. This allows for certain common functionalities to be performed on all objects, such as accessing the ToString() method or checking for equality using Equals().
Conclusion
The root class in .NET is represented by the System.Object class. It serves as the base for all classes in the .NET framework, providing essential methods and properties that are inherited by all other classes. Understanding the significance of the root class is crucial for effectively utilizing common functionalities and behaviors available to all objects in the .NET ecosystem.
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#