Rounding to 2 Decimal Places
In C#, you can round a number to two decimal places using various methods and techniques. One of the common ways to achieve this is by using the Math.Round() method. Here's how you can do it with examples:
Using Math.Round() method
The Math.Round() method is used to round a decimal number to the nearest integer or specified number of decimal places. To round a number to two decimal places, you can use the overload of Math.Round() that accepts a second argument specifying the number of decimal places.
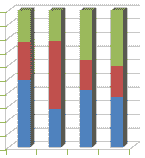
In this example, the variable number contains the value 3.14159. By using Math.Round(number, 2), the number is rounded to two decimal places, resulting in 3.14.
Using String Formatting
Another approach to round a number to two decimal places is by using string formatting:
In this example, the string.Format() method is utilized with the format specifier "{0:0.00}" to round the number to two decimal places. The formatted result is "5.68".
Using ToString() method with format
Alternatively, you can achieve the same result using the ToString() method with format specifier:
In this example, the ToString() method with the format "0.00" is employed to round the number to two decimal places, yielding "7.12".
Conclusion

Choose the method that best suits your coding style and requirements. Keep in mind that rounding numbers can lead to potential inaccuracies due to the nature of floating-point representations. If precise decimal rounding is critical, consider using the decimal data type instead of double.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Throwing Exceptions - C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#