Throwing Exceptions - C#
Exceptions are specialized objects that encapsulate irregular circumstances, such as instances when an application encounters out-of-memory conditions, fails to open a file, or encounters illegal type casting attempts. These descriptive exception objects are generated and subsequently raised using the "throw" keyword. Employing a "throw" statement within a catch block allows for the alteration of the resulting exception.
Throwing and Catching Exceptions
It is essential to exercise caution in throwing exceptions, reserving their use solely for unexpected or invalid occurrences that impede a method from executing its regular functionality. With the "throw" keyword, any type of Throwable object can be thrown, causing an interruption in the method's execution flow. Subsequent code beyond the throw statement will not be executed unless the thrown exception is appropriately handled.
C#Catch and throw Exception

You can re-throw a caught exception using the "throw" statement in C#. This practice allows for the propagation of the same exception to an upper-level catch block or calling method, providing more comprehensive error information during debugging and enhancing the overall robustness of the codebase.
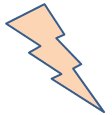
When re-throwing an exception, it is considered good programming practice to augment the exception with additional information. This could involve appending custom error messages, stack traces, or any other relevant data that aids in identifying the root cause of the exception. By enriching the exception with pertinent details, developers and maintainers can gain valuable insights into the error's origin, facilitating effective troubleshooting and resolution of issues.
C#throw; and throw ex;
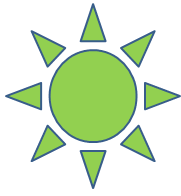
Exceptions indeed have a property called StackTrace, which holds information about the methods on the current call stack, including the file name and line number where the exception was thrown for each method. This property provides valuable debugging information that helps developers understand the sequence of method calls leading to the exception.
When an exception is thrown and caught, the stack trace can be preserved using the "throw" statement alone. This means that you can re-throw the caught exception without losing the original stack trace. By re-throwing the exception in this manner, you ensure that the original exception information, including the call stack, is retained, allowing for better debugging and error analysis.
Text
throw ex;When you use "throw ex" to re-throw an exception in .NET, the original stack trace is indeed lost, and the exception's stack trace starts from the point of the re-throw. This can lead to challenges in debugging applications as the original context of the exception is lost.
To maintain the original stack trace while re-throwing an exception, it is essential to use "throw;" without specifying the exception object. This allows the original exception to propagate up the call stack with its complete stack trace intact, ensuring better traceability and debugging capabilities.
Conclusion
By using "throw;" instead of "throw ex;", you ensure that the original exception's stack trace remains intact, making it considerably easier to debug the application and obtain appropriate trace messages.
- What is the root class in .Net
- How to set DateTime to null in C#
- How to convert string to integer in C#
- What's the difference between String and string in C#
- What is the best way to iterate over a Dictionary in C#?
- How to convert a String to byte Array in c#
- Detecting arrow keys in winforms C# and vb.net
- how to use enum with switch case c# vb.net
- Passing Data Between Windows Forms C# , VB.Net
- How to Autocomplete TextBox ? C# vb.net
- Autocomplete ComboBox c# vb.net
- How to convert an enum to a list in c# and VB.Net
- How to Save the MemoryStream as a file in c# and VB.Net
- How to parse an XML file using XmlReader in C# and VB.Net
- How to parse an XML file using XmlTextReader in C# and VB.Net
- Parsing XML with the XmlDocument class in C# and VB.Net
- How to check if a file exists in C# or VB.Net
- What is the difference between Decimal, Float and Double in .NET? Decimal vs Double vs Float
- How to Convert String to DateTime in C# and VB.Net
- How to Set ComboBox text and value - C# , VB.Net
- How to sort an array in ascending order , sort an array in descending order c# , vb.net
- Convert Image to Byte Array and Byte Array to Image c# , VB.Net
- How do I make a textbox that only accepts numbers ? C#, VB.Net, Asp.Net
- What is a NullReferenceException in C#?
- How to Handle a Custom Exception in C#
- Difference between string and StringBuilder | C#
- How do I convert byte[] to stream C#
- Remove all whitespace from string | C#
- How to remove new line characters from a string in C#
- Remove all non alphanumeric characters from a string in C#
- What is character encoding
- How to Connect to MySQL Using C#
- How convert byte array to string C#
- What is IP Address ?
- Run .bat file from C# or VB.Net
- How do you round a number to two decimal places C# VB.Net Asp.Net
- How to break a long string in multiple lines
- How do I encrypting and decrypting a string asp.net vb.net C# - Cryptography in .Net
- Type Checking - Various Ways to Check datatype of a variable typeof operator GetType() Method c# asp.net vb.net
- How do I automatically scroll to the bottom of a multiline text box C# , VB.Net , asp.net
- Difference between forEach and for loop
- How to convert a byte array to a hex string in C#?
- How to Catch multiple exceptions with C#