How and Why to Use Delegates in C#
A delegate is a type in C# that represents a reference to a method. Delegates provide a way to encapsulate a method, allowing it to be passed as an argument to other methods or stored as a field or property. It is a type in C# that represents a reference to a method. Also, it provide a way to encapsulate a method, allowing it to be passed as an argument to other methods or stored as a field or property.
A delegate type is defined using the delegate keyword and specifies the signature of the method it references, including the return type and any input parameters. Once a delegate type is defined, you can create a delegate instance that references a specific method by using the new keyword and passing in the name of the method or a lambda expression. Delegates can be invoked using the () operator, just like regular method calls. When a delegate is invoked, the referenced method is called with the arguments provided to the delegate.
Why do you need delegates in C#
Here are some reasons why delegates are useful in C#:
- Event Handling: One of the primary uses of delegates in C# is for event handling. By defining a delegate type that matches the signature of an event handler method, events can be raised and handled in a type-safe and efficient manner.
- Callback Mechanisms: Delegates provide a way to implement callback mechanisms, which enable a method to call back to a specified method or methods when a certain condition is met.
- Separation of Concerns: Delegates can be used to separate concerns in a program by allowing a method to be defined separately from the code that calls it. This can make code more modular, reusable, and easier to maintain.
- Asynchronous Programming: Delegates can be used to implement asynchronous programming patterns, such as the Asynchronous Programming Model (APM) and the Task-based Asynchronous Pattern (TAP), which enable long-running operations to be performed without blocking the calling thread.
- Functional Programming: Delegates are an important feature of functional programming in C#, as they enable the passing of functions as arguments to other functions, which is a fundamental concept in functional programming.
Declare a delegate
Follwoing is an example of how to declare a delegate named ArithmeticOperation:
In the above code, the delegate type ArithmeticOperation is declared with two int parameters and a return type of int. This delegate type can be used to represent any method that takes two int parameters and returns an int.
You can use this delegate to reference any method that matches this signature. For example, you can define a method named Add that adds two numbers and returns the result, and assign it to the delegate like this:
In the above code, the Add method is defined to take two int parameters and return their sum.
Instantiate a delegate
Following is an example of how to instantiate a delegate using the above ArithmeticOperation example:
In the above code, an instance of the ArithmeticOperation delegate is created using the new keyword and assigned to the Add method. This means that the operation delegate now refers to the Add method.
Invoke a delegate
Following is an example of how to invoke a delegate using the ArithmeticOperation example:
In the above code, the operation delegate is assigned to the Add method, which takes two int parameters and returns their sum. The Invoke method is called on the delegate instance, with two arguments, to compute the result.
Also, you can also invoke the delegate directly by calling it like a method, like this:
This is a shorthand notation for invoking the delegate and is equivalent to calling the Invoke method.
Delegates in C# | Example
Following is an example that demonstrates the use of delegates in C#:
Suppose you have a simple program that needs to perform an arithmetic operation on two numbers. Instead of hard-coding the operation, you want to provide a way for the user to specify the operation at runtime. To accomplish this, you can use a delegate to encapsulate the operation and pass it as an argument to the method that performs the arithmetic calculation.
In the above example, you define a delegate type called ArithmeticOperation that takes two integers and returns an integer. You then declare a delegate instance called operation that will reference the appropriate arithmetic method based on the user's input.
In the Main method, you prompt the user for two integers and an arithmetic operation. You then use a switch statement to assign the appropriate arithmetic method to the operation delegate. Finally, you invoke the delegate to perform the arithmetic operation and output the result to the console.
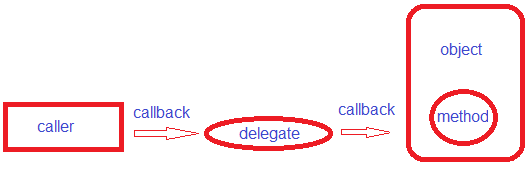
By using a delegate in this way, you are able to encapsulate the arithmetic operation and pass it as an argument to the method that performs the calculation, which provides a flexible and extensible solution to our problem.
Types of delegates in C#?
In C#, there are two main types of delegates:
- Singlecast Delegates
- Multicast Delegates
Singlecast Delegates:
Singlecast delegates are delegates that can reference a single method at a time. When a method is assigned to a singlecast delegate, any previous method references are overwritten. Singlecast delegates are declared using the delegate keyword followed by the delegate signature.
In the above code, MyDelegate is a singlecast delegate that takes two integers as arguments and returns void. The delegate instance del is assigned to the method MyMethod.
Multicast Delegates:
Multicast delegates are delegates that can reference multiple methods at the same time. When a method is assigned to a multicast delegate, it is added to a list of methods that the delegate will invoke. Multicast delegates are declared using the delegate keyword followed by the delegate signature, and the + or - operators are used to add or remove method references from the delegate.
In the above code, MyDelegate is a multicast delegate that takes two integers as arguments and returns void. The delegate instance del is initially set to null, and two methods, MyMethod1 and MyMethod2, are added to the delegate using the + operator.
When a multicast delegate is invoked, it will invoke all the methods in its invocation list in the order in which they were added. If any of the methods throw an exception, the remaining methods in the invocation list will still be called.
In addition to these main types of delegates, C# also supports generic delegates, which are delegates that can be parameterized with a type argument, and anonymous delegates, which are delegates that are defined inline without being assigned to a named delegate variable.
Generic Delegates in C#?
In C#, a generic delegate is a delegate that can be used to represent any method that matches its signature, regardless of the method's return type or parameter types. This allows you to create more flexible and reusable code, as you can use the same delegate type to reference multiple methods with different signatures.
In C#, the two primary generic delegate types are Action and Func.
Action:
This is a generic delegate type that represents a method that takes zero or more parameters and returns void. The number of parameters can range from zero to sixteen, depending on the number specified in the delegate type. For example, Action
Func:
This is a generic delegate type that represents a method that takes zero or more parameters and returns a value. The last parameter of the method represents the return type. Like Action, the number of parameters can range from zero to sixteen, depending on the number specified in the delegate type. For example, Func
How to use a generic delegate in C#:
In the above example, a generic delegate type CompareInts is defined that takes two int parameters and returns a bool value. A method LessThan is defined that matches the delegate's signature. An instance of the delegate is then created and assigned to the method. Finally, the delegate is called with two arguments and the result is stored in a variable.
Anonymous Delegates in C#
In C#, anonymous delegates are a way to define and create delegate instances without having to explicitly declare a named method. They allow you to define a block of code that can be executed when the delegate is invoked.
Anonymous delegates are useful when you need to pass a function as an argument to a method, but you don't want to define a named method for the function. They are often used in event handlers and with LINQ expressions.
How to create an anonymous delegate
In the above code, an anonymous delegate is created that takes an integer argument and writes a message to the console. The delegate is then assigned to a variable myDelegate and invoked with an argument of 10.
Note that you can also use lambda expressions to create anonymous delegates in C#.
This code does the same thing as the previous example, but uses a lambda expression to create the delegate instead of a traditional anonymous delegate syntax.
Conclusion:
C# delegates provide a powerful and flexible way to encapsulate and pass around methods as objects, enabling advanced programming patterns such as event-driven programming and callback mechanisms.
- C# Interview Questions (part-1)
- C# Interview Questions (part-2)
- C# Interview Questions (part-3)
- Difference between a Debug and Release build
- Difference between normal DLL and .Net DLL
- What is an Interface in C#
- Difference between Abstract Class and Interface in C#
- Difference between a thread and a process
- Differences between a control and a component
- Differences between Stack and Heap
- What is .Net Reflection
- Globalization and Localization | C#
- What is .Net serialization
- Difference between web service and .net remoting
- Difference between managed and unmanaged code
- Difference between Shallow copy and Deep copy
- Use of System.Environment Class
- What is the difference between private and shared assembly?
- Does the .NET have in-built support for serialization?
- How to properly stop the Thread in C#?
- Why are there five tracing levels in System.Diagnostics.TraceSwitcher?
- Why is XmlSerializer so slow?
- How many types of Jit Compilers?