Stack vs Heap Memory Allocation
Memory management in programming languages, two primary memory regions play distinct roles: the stack and the heap. The stack is specifically employed for static memory allocation, while the heap serves as the field of dynamic memory allocation. Both these memory regions reside within the computer's Random Access Memory (RAM) and perform essential functions in managing data storage.
Stack
Variables allocated on the stack are stored directly to the memory and access to this memory is very fast, and it's allocation is dealt with when the program is compiled. When a function or a method calls another function which in turns calls another function etc., the execution of all those functions remains suspended until the very last function returns its value. The stack is always reserved in a LIFO order, the most recently reserved block is always the next block to be freed. This makes it really simple to keep track of the stack, freeing a block from the stack is nothing more than adjusting one pointer.
Heap
Variables allocated on the heap undergo memory allocation at runtime, which leads to relatively slower access to this allocated memory. However, the heap size is not restricted by a fixed limit, as it is dependent on the available virtual memory. Unlike the stack, elements in the heap do not possess interdependencies and can be accessed randomly at any point in time. The heap allows for flexible allocation and deallocation of memory blocks, enabling developers to allocate a block when needed and free it when it is no longer required. This dynamic nature of the heap, however, introduces greater complexity in managing the allocation and deallocation of memory, as it requires careful tracking of which portions of the heap are currently in use and which ones are available for allocation.
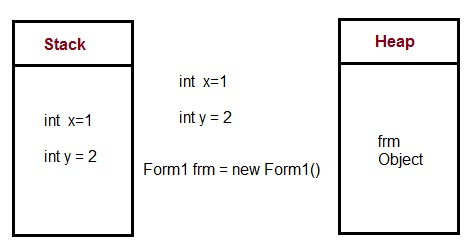
Choosing Between Stack and Heap
The stack is suitable for situations where the amount of data to be allocated is known in advance and can be determined before compile time. It is preferable for relatively smaller amounts of data. On the other hand, the heap is more appropriate when the exact amount of data needed at runtime is uncertain or when a large quantity of data needs to be allocated.
Using the stack is advantageous when you have a fixed size requirement and want to maximize efficiency, as accessing data from the stack is faster due to its LIFO structure. It provides a straightforward approach for managing memory and is well-suited for managing function calls and local variables.
In contrast, the heap offers flexibility in memory allocation, allowing for dynamic allocation and deallocation of data during runtime. It is beneficial when the size of data is unknown beforehand or when you need to allocate a significant amount of memory for larger data structures, such as objects, arrays, or complex data sets.
Choosing between the stack and the heap depends on the specific requirements of your program and the nature of the data being handled. Understanding the characteristics and trade-offs of each memory region helps in making informed decisions for efficient memory management in your application.
Memory Allocation in Multi-threaded Environments
In a multi-threaded scenario, each individual thread operates with its own distinct and autonomous stack, ensuring thread-specific memory management. However, all threads within the application share a common heap, which is responsible for application-wide memory allocation. The stack is intricately tied to exception handling and the execution of threads, making it a crucial aspect to consider in these contexts.
By contrast, the heap pertains to the broader scope of the entire application, encompassing memory allocation requirements that transcend individual threads. Understanding the distinctions between stack and heap is vital for effective exception management and seamless execution of threads within a multi-threaded environment.
Key differences between the Stack and Heap
Here are the key differences between the stack and heap in terms of memory allocation:
Stack:
- Memory Allocation: The stack is used for static memory allocation, where memory is allocated at compile time.
- Memory Management: Memory on the stack is automatically managed by the system, with allocation and deallocation handled in a last-in-first-out (LIFO) order.
- Speed: Accessing data from the stack is faster, as it involves simple pointer adjustments.
- Size Limit: The stack size is usually limited and determined by the system, and it is typically smaller compared to the heap.
- Memory Usage: The stack is used for storing local variables, function calls, and program execution context.
- Thread-specific: Each thread in a multi-threaded environment has its own independent stack.
Heap:
- Memory Allocation: The heap is used for dynamic memory allocation, where memory is allocated at runtime.
- Memory Management: Memory on the heap must be manually managed by the programmer, including explicit allocation and deallocation.
- Speed: Accessing data from the heap is relatively slower, as it involves indirect memory addressing.
- Size Limit: The heap size is not limited by a fixed size and can grow as long as the available virtual memory allows.
- Memory Usage: The heap is used for storing objects, arrays, and dynamically allocated data structures.
- Shared Memory: The heap is shared among all threads in a multi-threaded application.
- Flexibility: The heap provides flexibility in allocating and deallocating memory blocks as needed during runtime.
Conclusion
The stack and heap differ in their memory allocation approach, management, speed, size limits, and usage, with the stack being thread-specific and efficient for small, predictable memory needs, while the heap offers flexibility for dynamic allocation of larger memory blocks shared among threads.
- C# Interview Questions (part-1)
- C# Interview Questions (part-2)
- C# Interview Questions (part-3)
- Difference between a Debug and Release build
- Difference between normal DLL and .Net DLL
- What is an Interface in C#
- Difference between Abstract Class and Interface in C#
- Difference between a thread and a process
- Delegates in C# with Examples
- Differences between a control and a component
- What is .Net Reflection
- Globalization and Localization | C#
- What is .Net serialization
- Difference between web service and .net remoting
- Difference between managed and unmanaged code
- Difference between Shallow copy and Deep copy
- Use of System.Environment Class
- What is the difference between private and shared assembly?
- Does the .NET have in-built support for serialization?
- How to properly stop the Thread in C#?
- Why are there five tracing levels in System.Diagnostics.TraceSwitcher?
- Why is XmlSerializer so slow?
- How many types of Jit Compilers?