Java if-else statement
In Java, the "if-else" statement plays a crucial role in controlling the flow of program execution based on specific conditions. This statement allows developers to determine whether a particular block of code should be executed or not, depending on the evaluation of a condition.
The fundamental concept behind the "if-else" statement is to execute a specific block of code if a given condition evaluates to true. If the condition is false, an alternative block of code specified by the "else" clause is executed instead. This construct provides a way to make decisions and perform different actions based on the outcome of a condition.
Java if statement
The "if" statement in Java is a control flow statement that allows you to execute a block of code conditionally based on the result of a Boolean expression.
Syntax:The expression is a boolean expression that returns either true or false. If the expression evaluates to true, the code inside the set of curly braces {...} will be executed, and if it evaluates to false, the code inside the curly braces will be skipped.
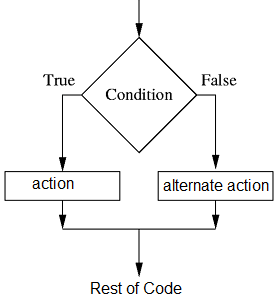
Example:
Java if-else statement
The "if-else" statement in Java is an extension of the "if" statement that allows you to specify an alternate block of code to be executed if the expression is false.
Syntax:The expression is a boolean expression that returns either true or false. If the expression evaluates to true, the code inside the first set of curly braces {...} will be executed, and if it evaluates to false, the code inside the second set of curly braces {...} will be executed.
Example:Multiple if-else statements in Java
You can also chain multiple if-else statements together to handle multiple conditions:
Syntax:In the above case, only the first expression that evaluates to true will have its corresponding code block executed, and all subsequent expressions and code blocks will be skipped.
Example:Nested if-else statement in Java
A "nested if" statement in Java is an " if" statement that is contained within another "if" statement. The basic idea behind a nested "if" statement is to test multiple conditions in a single control flow statement.
Syntax:In the above example, the first "if" statement tests the condition represented by expression1. If expression1 evaluates to true, the code inside the first set of curly braces {...} will be executed. Within this code block, there is another "if" statement that tests the condition represented by expression2. If expression2 also evaluates to true, the code inside the second set of curly braces will be executed.
Example:Nested "if" statements can be useful when you need to test multiple conditions in a single control flow statement. For example, you might use a nested "if" statement to check if a variable is within a certain range and take different actions based on whether the variable is greater than, less than, or equal to a certain value.
Common uses of the "if-else" statement in Java
- Conditional execution: The most common use of the "if-else" statement is to conditionally execute a block of code based on the result of a Boolean expression. For example, you might want to execute a block of code that performs a calculation only if a certain variable has a specific value.
- Decision making: The "if-else" statement can also be used to make decisions in your code based on different conditions. For example, you might use an "if-else" statement to determine which of two different actions to take based on the value of a variable.
- Error checking: The "if-else" statement can also be used to check for errors or invalid inputs in your code. For example, you might use an "if-else" statement to validate user input and display an error message if the input is not valid.
- Multiple branches: The "if-else" statement can also be used to handle multiple branches of execution based on different conditions. For example, you might use multiple "if-else" statements to handle different cases in a switch statement.
Alternative of if statement in Java
There are alternatives to the "if" statement in Java that can be used to control the flow of execution of a program based on certain conditions. Following are some of the most common alternatives:
Switch statement:
The "switch" statement is an alternative to the "if-else" statement that allows you to execute different blocks of code based on the value of a single expression. The "switch" statement is often used when you need to handle multiple cases for the same expression. For example, you might use a "switch" statement to handle different menu options in a program.
Ternary operator:
The ternary operator is a compact alternative to the "if-else" statement that allows you to write simple conditional expressions in a single line of code. The ternary operator takes the form expression1 ? expression2 : expression3, where expression1 is the condition, expression2 is the value to be returned if the condition is true, and expression3 is the value to be returned if the condition is false.
Conditional operator:
The conditional operator is another alternative to the "if-else" statement that allows you to write simple conditional expressions in a single line of code. The conditional operator takes the form expression1 ?: expression2, where expression1 is the condition and expression2 is the value to be returned if the condition is false.
These alternatives can provide a more concise, efficient, and readable way to control the flow of execution in your program, depending on the specific requirements of your code. It's important to choose the right alternative for your use case based on the desired behavior, performance, and readability of your code.
Conclusion
The correct syntax and indentation when using nested "if" statements, as well as to choose appropriate expressions that evaluate to true or false in order to produce the desired behavior in your code. Additionally, it's a good idea to be mindful of the complexity and readability of your code, as nested "if" statements can quickly become difficult to understand if they are not well organized and properly indented.