Java break and continue statement
In certain instances, it becomes advantageous to bypass specific statements within a loop or abruptly terminate the loop without evaluating the test expression. To accomplish this in Java, we can employ break statements. It is worth noting that unlike certain programming languages such as C and C++, Java does not include a Go To statement. Although the Java keyword list includes the "goto" keyword, it is marked as "not used."
The break statement serves as a powerful tool for control flow management. By incorporating break statements strategically within loops, we can effectively interrupt the execution and proceed to the subsequent statement or block of code outside the loop. This feature allows for enhanced flexibility and fine-grained control over loop iteration
Break statement
The break statement in Java finds its utility in conjunction with conditional switch statements, as well as the do, for, and while loop statements. Whenever a break statement is encountered within a loop, the loop's execution is promptly terminated, and program control seamlessly transitions to the subsequent statement following the loop.
This behavior of the break statement allows for precise control over the flow of program execution. By strategically placing break statements within loops, developers can promptly exit the loop and proceed to the next line of code, bypassing any remaining iterations or statements within the loop body.
It is important to recognize that the break statement acts as a powerful mechanism for altering the natural progression of program flow. Its ability to terminate loops prematurely offers flexibility and allows for efficient handling of specific conditions or scenarios.
Syntax: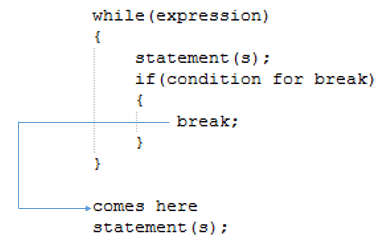
As depicted in the aforementioned image, the break statement serves to abruptly terminate the execution of the nearest enclosing loop or conditional statement in which it is present. Following the execution of the break statement, program control seamlessly transfers to the statement that follows the conclusion of the enclosing loop or conditional statement, if such a statement exists.
This behavior allows for precise control over program flow and provides a means to exit loops or conditional statements at specific points based on certain conditions or requirements. By strategically placing break statements, developers can effectively control the execution path and navigate to subsequent statements or code blocks as needed.
Example:In the above program, when cnt==3, the break statement executed and immediately terminated the while loop and the program control resumes at the next statement.
break statement in for loop
Continue statement
The continue statement in Java operates similarly to the break statement, but with a distinct purpose. Instead of terminating the loop, the continue statement forces the next iteration of the loop to take place, effectively skipping the remaining code within the loop for that iteration.
The continue statement finds its primary use within loop structures. When encountered inside a loop, whether conditionally or unconditionally, the continue statement redirects program control to the next iteration of either the current loop or an enclosing labeled loop.
Syntax: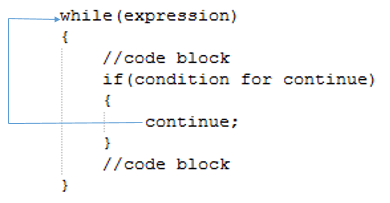
As seen in the above image, the continue statement ends current flow of execution and control directly jumps to the beginning of the loop for next iteration.
By utilizing the continue statement strategically, developers can control the flow of execution within loops and skip certain iterations based on specific conditions or requirements. This capability allows for fine-grained control over loop logic and the ability to selectively process or exclude certain iterations.
Example:In the baove program, we can see in the output the 3 is missing. It is because when cnt==3 the loop encounter the continue statement and control go back to start of the loop.
continue statement in for loop
Conclusion
It is important to note that the continue statement operates within the scope of the loop it is present in, either the current loop or an enclosing labeled loop. This targeted control flow manipulation enables developers to optimize program execution and streamline the processing of loop iterations.