Java throw exception
The throw keyword is used to throw an exception object, which represents an error condition. The type of the exception object can be any class that extends the java.lang.Throwable class.
Exceptions are objects representing error conditions that are raised or "thrown" during program execution.
Using throw keyword in Java
Java provides a mechanism for handling exceptions, which allows you to write code to detect and respond to exceptions when they occur. Exceptions can be thrown by the Java runtime system, or they can be explicitly thrown by your code.
Following is an example of how to throw an exception in Java:
In the above example, the throw statement is used to explicitly throw an exception of type Exception with the message "This is an exception". The exception is caught by the catch block and the message of the exception is printed.
Checked and unchecked exception when throwing?
There is a difference in checked and unchecked exceptions when throwing them.
Checked exceptions, such as IOException or SQLException, must be declared in the method signature using the throws keyword. If a method throws a checked exception and the exception is not caught and handled within the method, the method must declare the exception using the throws keyword. If a method does not handle the exception, it must pass the exception on to its calling method.
Unchecked exceptions, such as ArrayIndexOutOfBoundsException or NullPointerException, do not need to be declared in the method signature using the throws keyword. You can throw them without declaring them, and the Java compiler will not issue an error.
Throwing Checked Exception
Checked exceptions are exceptions that are required to be declared in the method signature using the throws keyword. This means that if a method throws a checked exception and the exception is not caught and handled within the method, the method must declare the exception using the throws keyword.
Following is an example of throwing a checked exception using the throw keyword:
In the above example, the readFile method declares that it might throw a FileNotFoundException using the throws keyword. The throw statement is used to throw the exception, which is caught and handled in the main method using a try/catch block. The message associated with the exception is printed to the console.
Throwing Unchecked Exception
Unchecked exceptions are exceptions that do not need to be declared in the method signature using the throws keyword. You can throw them without declaring them, and the Java compiler will not issue an error.
Following is an example of throwing an unchecked exception using the throw keyword:
In the above example, the divide method performs a division operation and checks whether the divisor is zero. If the divisor is zero, the method throws an ArithmeticException using the throw statement. The exception is caught and handled in the main method using a try/catch block, and the message associated with the exception is printed to the console.
Throwing User-defined Exception
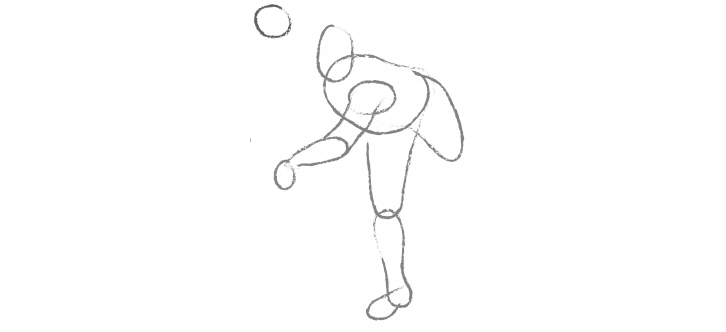
It's also possible to create your own custom exceptions by defining a new exception class that extends the Exception class or one of its subclasses. For example:
Then you can throw instances of your custom exception just like any other exception:
It's important to note that it's generally a good practice to catch specific exceptions, rather than catching the generic Exception type. This allows you to handle different types of exceptions differently and provides more information about the nature of the error.
Using the throws keyword in Java
The throws keyword in Java is used to indicate that a method might throw an exception. The throws keyword is used in the method signature to declare that a particular exception might be thrown by the method.
When a method declares that it might throw an exception using the throws keyword, the caller of the method is responsible for either catching the exception and handling it, or declaring that the method might throw the exception and passing the responsibility of handling it up the call stack to the next higher level.
In the above example, the readFile method declares that it might throw a FileNotFoundException using the throws keyword in the method signature. The throw statement is used to throw the exception, which is caught and handled in the main method using a try/catch block. The message associated with the exception is printed to the console.
Conclusion
It's important to use the throws keyword appropriately when declaring methods that might throw exceptions, and to catch and handle those exceptions in a way that ensures the stability and correct behavior of your program.