UnhandledPromiseRejectionWarning
The Promise object serves as a representation of the future outcome of an asynchronous operation, either successful or failed, along with its resulting value. The Promise.reject() method produces a Promise object that is intentionally rejected with a specified reason. If a JavaScript Promise doesn't have a rejection handler and gets rejected, the unhandledrejection event is emitted to the script's global scope, usually the window object or a Worker, highlighting an unhandled rejection scenario.
UnhandledPromiseRejectionWarning
The UnhandledPromiseRejectionWarning is typically triggered due to either throwing an error within an async function without proper error handling using a catch block, or by failing to handle a rejected promise with the .catch() method. When a promise is rejected without being caught, it resembles an unhandled exception that propagates upwards to the application's main entry point, prompting the root error handler to generate this warning. This commonly occurs within async/await functions, but there is a straightforward solution to address it.
Rejected Promise
The initial idea behind promises was that a rejected promise could exist without an immediate catch handler attached to it. In the past, some browsers, like Firefox, would only raise warnings for uncaught rejection errors when a rejected promise without a rejection handler was eventually removed from memory through garbage collection. However, this approach was modified due to concerns about developers not reliably managing promise rejections. Consequently, the HTML specification was altered, mandating browsers to generate "UnhandledPromiseRejectionWarning" errors if a rejected promise lacks rejection handlers before the event loop returns control to the code.

In certain situations, you can avoid notifying the host environment about promise rejections by incorporating a rejection handler that is essentially unused. The rationale behind this approach is that by adding a placeholder rejection handler to a promise, the promise has a handler in place in case it gets rejected. Consequently, the host environment is notified that the promise now has a rejection handler, effectively suppressing unhandled rejection notifications. This strategy also allows for the ability to invoke both the then() and catch() methods multiple times on the same promise.
catch(...) to a Promise
In accordance with the ES6 specification, a promise encapsulates the state transitions of an asynchronous operation, manifesting in "pending," "fulfilled," or "rejected" states. The occurrence of an UnhandledPromiseRejectionWarning can be attributed to the necessity for every promise to address potential promise rejections. This involves incorporating a .catch(...) method to handle potential rejections. The warning can be mitigated by simply adding a .catch(...) clause to the promise, as illustrated in the following example.
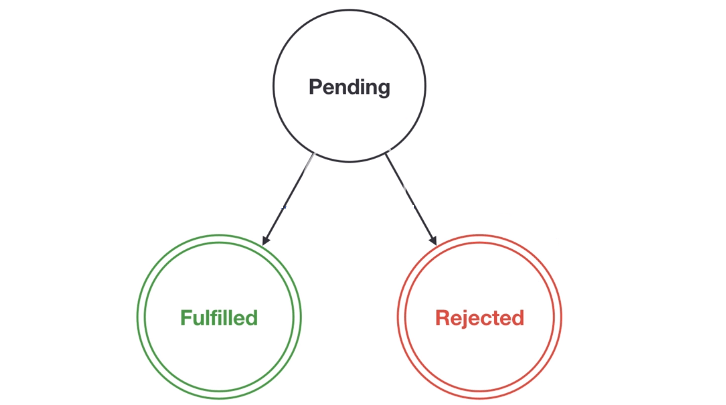
In the following code, the function promiseTest() will either resolve or reject a promise based on the value of a global variable globalVar:
The occurrence of the "UnhandledPromiseRejectionWarning" message can be perplexing, even when a .catch(...) clause is included in your code. This can transpire due to the sequence and arrangement of your asynchronous operations. For instance, in scenarios where the error is caught within a chained .catch(...) block after the initial unhandled rejection, the warning may still emerge. Ensuring comprehensive error handling, not just on individual promises but throughout the entire promise chain, is crucial to eliminate such warnings. An attentive and comprehensive approach to structuring your promise-related code can effectively preempt the emergence of these warnings.
The behavior you've described occurs due to how JavaScript handles promise chaining and error propagation. When a promise chain is structured in a way that errors are caught and handled separately for each promise, the JavaScript engine doesn't consider it as an unhandled promise rejection. In essence, by using individual .catch(...) blocks for each promise, you're explicitly indicating that you've accounted for error handling, which prevents the "UnhandledPromiseRejectionWarning" from being triggered. This approach enhances code clarity and mitigates potential warnings related to unhandled promise rejections.
Promise.all
It's a powerful tool in asynchronous programming that enables you to concurrently execute multiple promises and handle their results as a single unit. By providing an array of promises to Promise.all, you're effectively instructing JavaScript to wait until all of them have resolved, and then it returns a promise that resolves to an array of their resolved values. This mechanism is particularly useful when you have multiple independent asynchronous operations that need to be executed in parallel and their results need to be aggregated once they are all complete. It's a great way to enhance performance and manage multiple asynchronous tasks efficiently.
Conclusion
UnhandledPromiseRejectionWarning is a message generated by JavaScript when a promise is rejected but no explicit rejection handler (.catch() or try-catch) is attached to it. This warning indicates that a rejected promise doesn't have proper error handling, which can potentially lead to unhandled errors in your code. To resolve this warning, make sure to add appropriate error handling mechanisms to your promises, either by attaching .catch() or using try-catch blocks.