Read text file using Python
In Python, you can read a text file using the built-in open function. Here's an example of how to read the contents of a text file and store it in a string:
Read the text file line by line in Python
The read method reads the entire contents of the file into a single string. If you want to read the file line by line, you can use a for loop:
Remove the line terminator
Each iteration of the loop retrieves the next line in the file, including the line terminator. You can use the strip method to remove the line terminator:
Reads the entire contents of a text file
The read method reads the entire contents of a file and returns it as a string. For example:
Reads a single line from a txt file
The readline method reads a single line from a file and returns it as a string. For example:
In the above example, the while loop continues to read lines from the file until readline returns an empty string, indicating the end of the file. The strip method is used to remove the line terminator from each line.
Steps for reading a txt file | Python
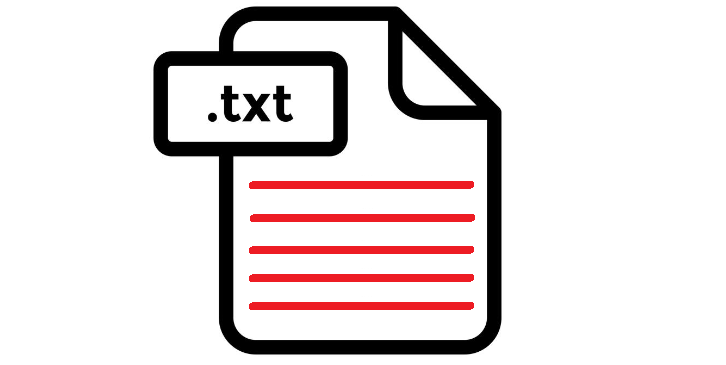
open() function
You can use the built-in open function to open a file and read its contents. You can specify the mode as "r" for reading and "w" for writing. The read method can be used to read the entire contents of the file into a string, or you can use a for loop to read the file line by line.
with statement
The with statement is a context manager that ensures that a file is properly closed after it's no longer needed. You can use the with statement in combination with the open function to read a file, as shown in the previous example.
file object
The file object is a built-in type in Python that represents an open file. You can use a file object to read a file by calling its read method, or you can use a for loop to read the file line by line.
read() method
The read method reads the entire contents of a file and returns it as a string.
These are some of the most common ways to read txt files in Python. You can choose the method that works best for your needs based on the size and structure of the file you want to read.
File access mode in Python
In Python, when you open a file using the open function, you can specify the mode in which the file should be opened. Here are the most commonly used file access modes:
- "r" (read mode): Open the file for reading. If the file does not exist, a FileNotFoundError is raised.
- "w" (write mode): Open the file for writing. If the file exists, its contents are truncated. If the file does not exist in the path, a new file is created.
- "a" (append mode): Open the file for writing, but instead of truncating its contents, new data is added to the end of the file. If the file does not exist in the path, a new file is created.
- "+" (read and write mode): Open the file for reading and writing. The file's pointer is positioned at the starting of the file.
In most cases, you will use either the "r" or "w" mode when working with text files, and "rb" or "wb" when working with binary files. The other modes can be used in specific cases where you need more control over the way the file is opened.
Conclusion
Reading text files in Python involves using the open() function to open the file in read mode, followed by methods like read(), readline(), or readlines() to retrieve the content. Proper file closure with the close() method or utilizing the "with" statement is important for resource management.