Python Conditional Statements
Decision-making stands as a cornerstone within the scope of computer programming, embodying major significance. It necessitates developers to carefully delineate one or more conditions, subject to evaluation or testing by the program. Linked with these conditions are statements, poised for execution contingent upon their truth. Moreover, provisions for alternative executions, under the aegis of falsehood, can also be optionally defined. The Python programming language offers an array of decision-making constructs, furnishing a versatile toolkit for this purpose.
- if statements
- if....else statements
- if..elif..else statements
- nested if statements
- not operator in if statement
- and operator in if statement
- in operator in if statement
Python if statements
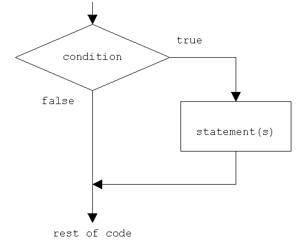
In Python, the "if" statement assesses the test expression enclosed within parentheses. Should the test expression resolve to a true value (nonzero), the statements housed within the "if" block are executed. Conversely, if the test expression evaluates to a false value (0), the statements within the "if" block are bypassed.
exampleWithin this program, two variables, namely 'x' and 'y', are instantiated. 'x' is initialized with the value 20, and 'y' with 10. Subsequently, the "if" statement scrutinizes the expression (x > y) for its veracity. In this specific instance, the evaluation x > y holds true, since 'x' assumes the value 20 while 'y' is set at 10. As a consequence, the program's control is directed into the body of the "if" block, leading to the printing of the message "X is bigger." In scenarios where the condition proves to be false, the program's flow proceeds beyond the confines of the "if" block.
Python if..else statements
The "else" statement serves the purpose of defining a code block that is to be executed when the condition within the "if" statement turns out to be false. As a result, the "else" clause guarantees the execution of a predetermined sequence of statements.
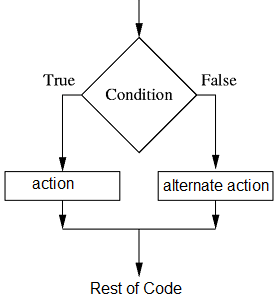
In the above code, the if stat evaluate the expression is true or false. In this case the x > y is false, then the control goes to the body of else block , so the program will execute the code inside else block.
if..elif..else statements
The elif is short for else if and is useful to avoid excessive indentation.
exampleIn the above case Python evaluates each expression one by one and if a true condition is found the statement(s) block under that expression will be executed. If no true condition is found the statement(s) block under else will be executed.
Nested if statements
In some situations you have to place an if statement inside another statement.
not operator in if statement
By using the "not" keyword, we possess the ability to alter the interpretation of expressions, enabling us to effectively reverse the outcome of an expression.
exampleYou can write same code using "!=" operator.
exampleand operator in if statement
The equivalent of "& & " is "and" in Python.
examplein operator in if statement
exampleConclusion
Python's "if" statements provide conditional execution by evaluating a test expression enclosed in parentheses. If the expression resolves to true, the statements within the associated block are executed; otherwise, alternative actions can be taken through "else" and "elif" clauses. The "not" keyword can be used to invert expressions, altering their logical meaning.