How can I get the data-id attribute | jQuery
A data attribute encompasses any attribute associated with an element that commences with "data-". jQuery offers a range of methods for interacting with HTML elements. To retrieve the value of a data attribute, such as "data-id", the jQuery attr() method can be employed, allowing straightforward access to the desired attribute's value for the specified HTML element.
The attr() method sets or returns attributes and values of the selected elements.

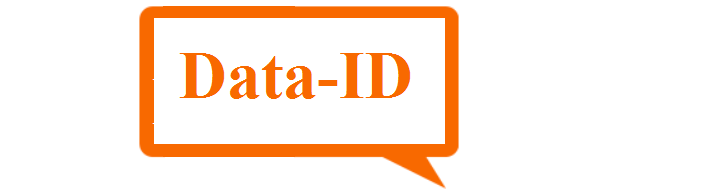
.data('attribute') method
If you are using a more recent version of jQuery (1.4.3 and above), the .data() method becomes available, enabling the attachment of data of diverse types to DOM elements in a manner that mitigates circular references and the potential memory leaks associated with them. This method ensures the safe management of data associated with elements, contributing to improved memory utilization and enhanced code reliability.
The .data() function expects and works only with lowercase attribute names.
Example
Conclusion
To access the data-id attribute using jQuery, you can employ the .attr("data-id") method for older versions or the .data("id") method for newer versions (1.4.3 and above). These methods facilitate the retrieval of the value assigned to the specified data attribute, enabling efficient interaction with the data associated with HTML elements.
- How to get input textbox value using jQuery
- JQuery Get Selected Dropdown Value on Change Event
- How to submit a form using ajax in jQuery
- How can I get the ID of an element using jQuery
- Open Bootstrap modal window on Button Click Using jQuery
- How to select an element with its name attribute | jQuery
- How to disable/enable submit button after clicked | jQuery
- How to replace innerHTML of a div using jQuery
- How to get the value of a CheckBox with jQuery
- How to wait 'X' seconds with jQuery?
- How to check if an enter key is pressed with jQuery
- How to allow numbers only in a Text Box using jQuery
- How to dynamically create a div in jQuery?