How to Create a DIV element using jQuery
jQuery offers several methods for dynamically adding a <div> element to the DOM, with append() and prepend() being two of the most straightforward options. The append() method inserts the new element as the last child of its parent, while the prepend() method adds the new element as the first child of its parent. These methods provide a convenient and efficient means to manipulate the structure of a webpage using jQuery.
jQuery prepend()
The jQuery prepend() method is an inherent function utilized to insert a specified content at the beginning of the chosen element. This approach is particularly useful for dynamically modifying the content and structure of HTML elements within a webpage using jQuery, providing a seamless way to enhance user interfaces and interactivity.
jQuery append()
The jQuery append() method is a built-in feature designed to insert an HTML element at the conclusion of the targeted element. This method proves advantageous for dynamically adding content to HTML elements within a webpage using jQuery, enabling developers to enhance user experiences and customize the structure of the page.
Create a DIV element | jQuery
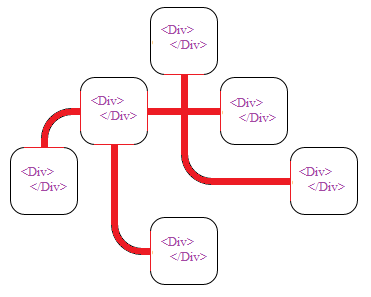

This is main Div
Conclusion
Creating a <div> element using jQuery can be achieved by employing methods like append() to add elements at the end, or prepend() to add them at the beginning of the selected element. Both methods facilitate dynamic content insertion, enhancing the structure and interactivity of a webpage by allowing developers to efficiently incorporate new <div> elements.
- How to get input textbox value using jQuery
- JQuery Get Selected Dropdown Value on Change Event
- How to submit a form using ajax in jQuery
- How can I get the ID of an element using jQuery
- Open Bootstrap modal window on Button Click Using jQuery
- How to select an element with its name attribute | jQuery
- How to get the data-id attribute using jQuery
- How to disable/enable submit button after clicked | jQuery
- How to replace innerHTML of a div using jQuery
- How to get the value of a CheckBox with jQuery
- How to wait 'X' seconds with jQuery?
- How to check if an enter key is pressed with jQuery
- How to allow numbers only in a Text Box using jQuery