How to select an element by name with jQuery
In jQuery, the selection of any specific element attribute is accomplished through the application of the [attribute_name=value] construct. This attribute-based selection mechanism empowers developers to precisely identify and manipulate elements based on specific attribute-value pairs. By utilizing this approach, developers are enabled to efficiently target and interact with elements that possess distinct attributes, enriching the precision and effectiveness of their code implementations.
It is a mandatory requirement to include quotes around the value.
Example
Also, you can use the jQuery attribute selector:
Multiple selction by attribute
You can select multiple element attribute by its name.

Using JavaScript
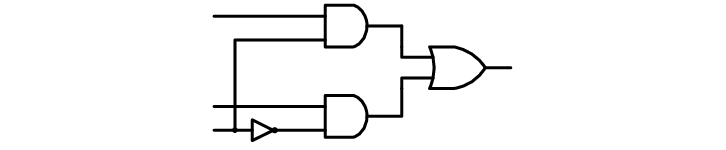
In JavaScript, the process of selecting an element's attribute can be achieved through the utilization of the getElementsByName() method. This method facilitates the retrieval of elements bearing a particular attribute name, presenting a straightforward approach to access and manipulate specific attributes within the context of web development.

jQuery element Selector
The jQuery element selector selects elements based on the element name.
You can select all ‹input› elements on a page like this:
When a user clicks on a button, all ‹input› elements will be hidden:
jQuery #id Selector
The jQuery #id selector employs the unique id attribute assigned to an HTML tag to precisely locate and identify a specific element within the DOM structure. This selector serves as an efficient means to singularly target elements based on their distinctive identifiers, enhancing the accuracy and efficiency of element manipulation.
To find an element with a specific id, write a hash character, followed by the id of the HTML element:
ExamplejQuery .class Selector
The jQuery .class selector finds elements with a specific class.
To find elements with a specific class, write a period character, followed by the name of the class:
ExampleConclusion
By specifying the attribute [name="username"], you can precisely target elements based on their name attribute using jQuery. This technique is particularly useful for form elements and other elements that require identification via the name attribute.
- How to get input textbox value using jQuery
- JQuery Get Selected Dropdown Value on Change Event
- How to submit a form using ajax in jQuery
- How can I get the ID of an element using jQuery
- Open Bootstrap modal window on Button Click Using jQuery
- How to get the data-id attribute using jQuery
- How to disable/enable submit button after clicked | jQuery
- How to replace innerHTML of a div using jQuery
- How to get the value of a CheckBox with jQuery
- How to wait 'X' seconds with jQuery?
- How to check if an enter key is pressed with jQuery
- How to allow numbers only in a Text Box using jQuery
- How to dynamically create a div in jQuery?