Get the ID of an Element using jQuery
The id property within the element interface embodies the identifier associated with the element, reflecting the globally recognized ID attribute. This identifier must be distinct and singular within a given document.
$('selector').attr('id') will return the id of the first matched element.ID Selector
Class Selector
If you want to get an ID of an element, let's say by a class selector, when an event was fired on that specific element, then the following will do the job:
More than one element ID
If your matched set contains more than one element, you can use the conventional .each loop to return an array containing each of the ids:

ID Selector ("#id")
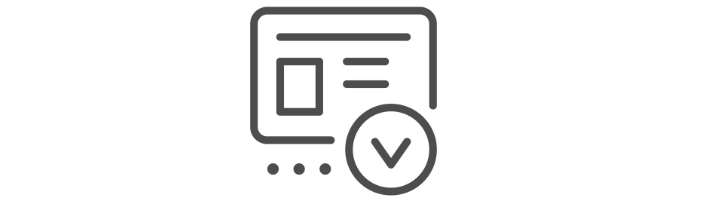
When you employ $("#something") in jQuery, it yields a jQuery object encapsulating the corresponding DOM element(s). Should you wish to retrieve the first matched DOM element directly, you can achieve this by using the get(0) method. This technique is particularly useful when you need to work with the actual DOM element instead of the jQuery object.
On this native element, you can call id, or any other native DOM property or function.
Or
Conclusion
When working with jQuery, obtaining the ID of an element involves using either the .attr("id") method or directly accessing the .id property. By utilizing these approaches, you can effortlessly retrieve the unique identifier assigned to an element within the DOM. Additionally, should you require the underlying DOM element itself, employing .get(0) after selecting an element with $("#something") enables direct access to the first matching element.
- How to get input textbox value using jQuery
- JQuery Get Selected Dropdown Value on Change Event
- How to submit a form using ajax in jQuery
- Open Bootstrap modal window on Button Click Using jQuery
- How to select an element with its name attribute | jQuery
- How to get the data-id attribute using jQuery
- How to disable/enable submit button after clicked | jQuery
- How to replace innerHTML of a div using jQuery
- How to get the value of a CheckBox with jQuery
- How to wait 'X' seconds with jQuery?
- How to check if an enter key is pressed with jQuery
- How to allow numbers only in a Text Box using jQuery
- How to dynamically create a div in jQuery?