Defaultdict in Python
Python defaultdict is a subclass of the dict class in Python's standard library. It overrides one method, __missing__, to provide a default value for a nonexistent key in the dictionary. The default value is specified as an argument when the defaultdict is created.
Syntax:
Here, default_value is the default value that will be returned when a key that doesn't exist in the dictionary is accessed.
Working with defaultdict
When you create a defaultdict, you specify a default factory function that is used to generate the default value for keys that haven't been set yet. The default factory function is called with no arguments and should return the default value for the dictionary.
In the above example, create a defaultdict named color_counts with a default factory function int. This means that if you try to access a key that doesn't exist in the dictionary, the default value 0 will be returned.
Then increment the counts for 'red', 'blue', and 'green'. Since these keys didn't exist in the dictionary initially, the default factory function is called to generate the default value of 0, and then the counts are incremented accordingly.
The resulting dictionary color_counts contains the keys 'red', 'blue', and 'green', each with its corresponding count. Note that since the default factory function is int, the values associated with the keys are integers.
Following are some examples of how to use defaultdict:
Creating a defaultdict with a list:
In the above example, the defaultdict is created with list as the default value. When the key 'red' is accessed, it is not yet in the dictionary, so the default value of an empty list [] is returned. Then append the values 100 and 200 to the list associated with the key 'red'. When the key 'blue' is accessed, it is also not yet in the dictionary, so the default value of an empty list [] is returned. Then append the value 300 to the list associated with the key 'blue'.
Creating a defaultdict with a lambda function:
In the above example, the defaultdict is created with a lambda function that returns the string unknown' ' as the default value. When the key 'r' is accessed, it is not yet in the dictionary, so the lambda function is called and returns 'unknown'. Then assign the value 'red' to the key 'r'. When the key 'b' is accessed, it is also not yet in the dictionary, so the lambda function is called and returns 'unknown'.
Creating a defaultdict with a set:
In the above example, the defaultdict is created with set as the default value. When the key 'red' is accessed, it is not yet in the dictionary, so the default value of an empty set {} is returned.
Dictionary Vs defaultdict
The main difference between defaultdict and a normal dictionary in Python is the way they handle missing keys.
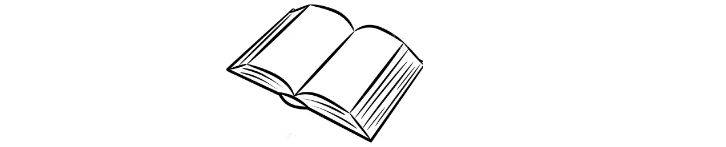
In a normal dictionary, if you try to access a key that doesn't exist in the dictionary, a KeyError is raised. You can use the get method or a try-except block to handle this error and provide a default value, but this requires extra code.
In contrast, defaultdict automatically creates a new key-value pair using a default factory function when a missing key is accessed. This can simplify your code and make it more readable.
Following is an example to illustrate the difference:
In the above example, create two dictionaries - normal_dict and default_dict. Then try to increment the value associated with the key 'red' in both dictionaries, but 'red' is not yet in normal_dict, so it raises a KeyError.
In contrast, when try to increment the value associated with the key 'red' in default_dict, a new key-value pair is created with a default value of 0, and then the value is incremented to 1.
Another difference between defaultdict and a normal dictionary is that the default factory function is specified when the defaultdict is created. This means that you can use different types of default values for different defaultdict instances, whereas a normal dictionary has a fixed behavior when it comes to missing keys.
Conclusion:
Python defaultdict is a useful tool for working with dictionaries because it simplifies the code needed to handle missing keys. Rather than needing to explicitly check if a key exists in the dictionary before accessing it, you can simply use the key and let defaultdict create a default value if necessary.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python