Reversing a List in Python
In Python, a list is a data structure used to store a collection of elements, which can be of different data types, such as integers, strings, and even other lists. Lists are ordered, mutable (i.e., you can change their contents), and allow duplicates.
There are several methods to reverse a Python list, and the following section explain each one in detail with examples:
Using the reverse() method
The easiest way to reverse a list in Python is to use the built-in reverse() method. This method modifies the original list in place, and does not return a new list.
Using slicing notation
Another way to reverse a list is to use slicing notation. Slicing allows you to extract a portion of the list, and by specifying the start and end indices, you can extract the entire list in reverse order.
Using the reversed() function
The reversed() function returns an iterator that generates the items of the original list in reverse order. You can use the list() function to convert the iterator into a list.
Using a loop
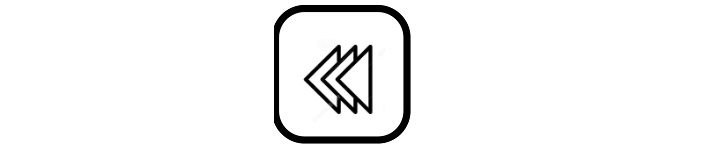
You can also reverse a list by iterating over it and appending each item to a new list in reverse order.
Using the list comprehension
List comprehension is a concise way to create a new list by iterating over an existing list. You can use list comprehension to reverse a list by iterating over it in reverse order.
Choose the method that fits your needs best.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python