Int Object is Not Iterable
The error message "int object is not iterable" occurs when you try to use an operation or function that requires an iterable object on a non-iterable object. In Python, an iterable is any object that can be looped over using a for loop or other methods that expect a sequence of values.
An integer value is not iterable, so you cannot use it in a for loop or with functions that expect an iterable as their argument. To fix this error, make sure that you are passing an iterable object to functions or operations that expect one.
'int' object is not iterable| situation
Following is an example situation where this error can occur:
Using the for loop on an integer:
An integer value is not iterable, so you cannot use it in a for loop.
How to fix: int' object is not iterable
Following are some solutions to fix this error:
Convert the integer to an iterable:
If you need to iterate over a single integer value, you can wrap it in a list or tuple to create an iterable:
Use a range() function:
If you want to iterate over a range of integer values, you can use the built-in range() function to generate an iterable sequence of numbers:
How to Ensure that the object being iterated?
Make sure that the object you are iterating over is actually iterable. If it's not, you will need to modify your code to work with an iterable object. For example, you cannot iterate over an integer using the for loop, so you will need to wrap it in an iterable container like a list or tuple.
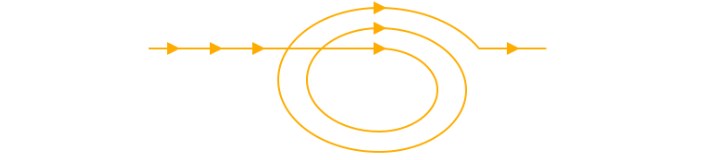
Check the type of the object being iterated over:
Double-check the type of the object you are trying to iterate over. If it's not an iterable, you will need to modify your code to work with an iterable object. For example, if you are trying to use the join() method on an integer, you will need to convert it to a string first.
Use a try-except block to handle the error:
If you are not sure if an object is iterable or not, you can use a try-except block to handle the error gracefully.
By using one or more of these solutions, you should be able to fix the "int object is not iterable" error and make your code work as expected.
What is an iterable object?
In Python, an iterable is any object that can be looped over using a for loop or other methods that expect a sequence of values. An iterable object returns an iterator object when the built-in iter() function is called on it. The iterator object can be used to get the next value in the sequence by calling the next() function on it. Examples of iterable objects in Python include lists, tuples, strings, dictionaries, sets, and generator functions.
Iterables are an essential concept in Python because they allow you to work with large datasets and process them efficiently. By using iterables and iterators, you can loop over large datasets without loading them all into memory at once, making your code more memory-efficient and faster.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python