Convert Decimal to Binary in Python
Here's a one-liner code to convert decimal to binary in Python using the built-in bin() function:
The bin() function converts the decimal number to a binary string with a prefix of "0b", so here use [2:] to slice off the first two characters of the string (i.e. the "0b" prefix) and return the rest of the string as the binary number.
Decimal and Binary
Decimal and binary are number systems used to represent numbers in computer programming. Decimal is a base-10 number system, which means it uses 10 digits (0-9) to represent all possible numbers. Binary is a base-2 number system, which means it uses only two digits (0 and 1) to represent all possible numbers.
There are a few different methods to convert decimal to binary in Python, and the following secion will go over each of them in detail below.
Using the built-in bin() function
The easiest way to convert a decimal number to binary in Python is to use the built-in bin() function. This function takes a decimal number as its argument and returns a string representing the binary equivalent of that number.
As you can see in the above code, the bin() function returns a string that starts with the prefix "0b". This prefix indicates that the string represents a binary number.
Using a loop and bitwise operators
If you don't want to use the bin() function, you can also convert a decimal number to binary using a loop and bitwise operators. This method involves repeatedly dividing the decimal number by 2 and keeping track of the remainders to build up the binary number.
In the above example, start by initializing an empty list binary_digits to hold the binary digits. Then enter a loop that continues as long as the decimal number is greater than zero. In each iteration of the loop, calculate the remainder of dividing the decimal number by 2, append that remainder to the binary_digits list, and then divide the decimal number by 2 using integer division (//).
Once the loop is finished, reverse the order of the binary digits in the binary_digits list (since built them up in reverse order) and join them together into a single string using the join() method.
Using the format() method
Another way to convert a decimal number to binary in Python is to use the format() method with the binary format specifier (b). This method takes a decimal number as its argument and returns a string representing the binary equivalent of that number.
In the above example, pass the decimal number and the string 'b' as arguments to the format() method. The 'b' format specifier tells Python to format the number as a binary string.
Using the bitwise shift operator >>
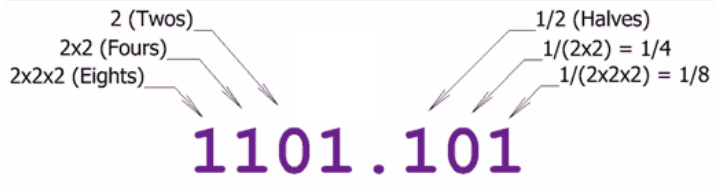
The bitwise shift operator >> is a binary operator in Python (and other programming languages) that shifts the bits of a number to the right by a specified number of positions. The operator is used in conjunction with an integer value, which specifies the number of bit positions to shift the number.
Following is an example code to convert a decimal number to binary using the bitwise shift operator (>>) in Python:
In the above code, start by initializing an empty list binary_digits to hold the binary digits. Then enter a loop that continues as long as the decimal number is greater than zero. In each iteration of the loop, append the least significant bit of the decimal number (i.e. the result of decimal_number & 1) to the binary_digits list, and then shift the decimal number one bit to the right using the right-shift operator (>>=).
Once the loop is finished, reverse the order of the binary digits in the binary_digits list (since built them up in reverse order) and join them together into a single string using the join() method. The resulting string is the binary representation of the original decimal number.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python