for loop in Java
In numerous situations within applications, there arises a need to execute a specific block of statements repeatedly. Loops serve as a fundamental construct in programming, enabling the execution of a code block multiple times. Among the loop types available in Java, the for loop proves particularly valuable for iterative operations, especially when working with arrays or requiring sequential processing.
The for loop provides a concise and structured approach to iterate over arrays, perform sequential operations, or execute a block of code a predetermined number of times. Its syntax consists of three essential components: initialization, condition, and increment/decrement.
Syntax:termination: defines the condition for running the loop (the code block).
increment: is executed each time after the loop (the code block) has been executed.
Within the for loop, the initialization step sets the initial state or starting point for the iteration. The condition defines the termination condition for the loop, determining whether the loop should continue or terminate. The increment or decrement step modifies the loop variable, ensuring progression through the loop iterations.
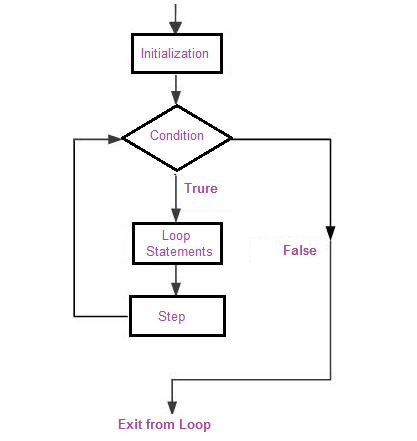
The for loop in Java follows a specific sequence of actions during its execution. It begins by initializing the loop control variable before entering the loop body. The loop then checks the condition against the current value of the control variable. If the condition evaluates to true, the loop statement is executed. After executing the loop body, the step statement is performed, modifying the control variable for each iteration.
For loops are particularly useful when the number of iterations is known in advance. They provide a concise and efficient way to express looping constructs, as they encapsulate the initialization, condition, and increment/decrement steps in a single line of code.
Example:The initializer declares and initializes a local loop variable, cnt, that maintains a count of the iterations of the loop. The loop will execute four(4) times because we set the condition cnt is less than or equal to counter.
The output of the code as follows :
Infinite Loop
In the Java for loop, all three components of the loop statement, namely initialization, condition, and increment/decrement, are optional. This flexibility allows developers to adapt the for loop to different scenarios and requirements.
If all three components are omitted, the loop becomes an infinite loop, meaning it will continue executing indefinitely until an external intervention is applied, such as terminating the program manually. This occurs because without a condition to evaluate, there is no mechanism to exit the loop naturally.
In the above code the loop will execute infinite times because there is no initialization , condition and steps .
To create an infinite loop intentionally, one can leave the conditional expression empty within the for loop declaration. This omission prevents any condition from becoming false, thus causing the loop to execute endlessly.
It is worth noting that infinite loops are generally undesirable in most scenarios, as they can lead to resource exhaustion and unresponsive programs. However, there are specific use cases where an infinite loop might be intentionally implemented, such as in server applications or real-time systems that require continuous execution.
Processing Arrays using Loops
An array serves as a data structure that allows for the storage of a collection of elements. When dealing with arrays, individually processing each element can be a cumbersome task. To simplify this process, the for loop provides an alternative form specifically designed for iterating through collections and arrays. This form enables developers to write more concise and readable loops.
By utilizing a for loop, developers can iterate through the elements of an array in a structured and efficient manner. A common approach involves using a loop variable to keep track of the index number as it iterates through the entire array. The following program uses the for to loop through the array:
Check with the following enhanced for loop is used to print the elements of the array.
In the above code, value of i is first initialised to arr[0] i.e. 2 and the body of the for loop is executed which will causes the integer 3 to be printed on the screen. Next arr[1] i.e. 4 is assigned to x and the body executed again. In this way the loop continues until all the elements are printed. Here, we can see the enhanced for loop is used to iterate through not just an array, but a collection in general.
Conclusion
The "for" loop in Java is a control structure used to repeat a block of code for a specified number of times or until a specific condition is met. It allows for efficient and concise iteration through arrays, collections, or any set of elements in Java programming.