Java Switch Statement
The switch statement in Java is a powerful control structure that facilitates the execution of various actions depending on different conditions. This construct is compatible with the byte, short, char, and int primitive data types, along with enumerated types, the String class, and specific wrapper classes like Character, Byte, Short, and Integer. It provides an efficient and structured way to handle multiple case scenarios within a program, enhancing code readability and maintainability.
Syntax:The switch expression in Java is evaluated only once, and its value is then compared with the values of each case. When a match is found, the corresponding block of code is executed. If there is no matching case, the program may proceed to the optional default clause, where associated statements will be executed. To ensure proper control flow, the optional break statement, attached to each case label, is used to exit the switch once the matched statement is executed. Omitting the break statement leads to continued execution of subsequent statements within the switch block.
Example:In the above case, inDay is the parameter to switch. Depends on switch parameter the day string is assigned the corresponding value. Here inDay=2, so the switch assign day="Monday".
switch..case on a String
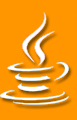
Switch statements with String cases were introduced in Java SE 7, which came approximately 16 years after their initial request. The reason for the delay was not explicitly stated, but it is believed to be associated with performance considerations. Implementing efficient String case handling in switch statements likely posed technical challenges and required careful optimization to ensure smooth execution and maintain Java's performance standards.
Switch statements based on integer types can be optimized to generate highly efficient code, allowing for faster execution and better performance. However, when using other data types, such as strings or custom objects, switch statements are limited in their optimization capabilities. In such cases, the switch statement may be compiled into a series of if() statements, which can lead to less efficient code execution.
Conclusion
Due to these optimization limitations, programming languages like C and C++ only permit switch statements to be used with integer types. This restriction ensures that the switch statement's efficiency is maintained, providing a more predictable and reliable performance for developers working with these languages.