How to open a Bootstrap modal window using jQuery
Opening a Bootstrap modal window using jQuery involves triggering the modal's display through a combination of HTML, Bootstrap classes, and jQuery event handling. Here's a comprehensive guide with examples:
HTML Structure
Set up the HTML structure for the modal and the trigger element that will initiate its opening. This can be a button, link, or any element you want to use as a trigger.
jQuery Event Handling
Use jQuery to handle the click event on the trigger element and programmatically open the modal.
In this example, clicking the "Open Modal" button triggers the click event handler associated with #openModalBtn. Within this handler, the $("#myModal").modal("show") command uses the Bootstrap modal method to display the modal window.

Full Source | jQuery
When you click the "Open Modal" button, the modal will appear with its designated content.
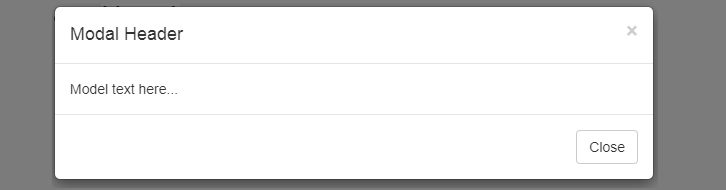
Due to how HTML5 defines its semantics, the autofocus HTML attribute has no effect in Bootstrap modals. To achieve the same effect, use some custom JavaScript:
This event fires immediately when the show instance method is called. If caused by a click, the clicked element is available as the relatedTarget property of the event.
Bootstrap $('#myModal').modal('show') is not working: why?
Most common reason for such problems are:
- Forgot to include jQuery library in the document.
- Included the Jquery library more than once in the document.
- The versions of your Javascript and Bootstrap does not match.
- Forgot to include bootstrap.js library in the document.
- The modal ‹div› is missing the modal class.
- # sign is missing in the Jquery selector.
- The id of the modal ‹div› is incorrect.
Conclusion
To open a Bootstrap modal window using jQuery, you first set up the HTML structure with a trigger element and the modal content. Utilizing jQuery, you attach a click event handler to the trigger element that invokes the .modal("show") method on the modal element, enabling the modal window to display interactively. This approach provides an elegant way to present additional content or interactions to users.
- How to get input textbox value using jQuery
- JQuery Get Selected Dropdown Value on Change Event
- How to submit a form using ajax in jQuery
- How can I get the ID of an element using jQuery
- How to select an element with its name attribute | jQuery
- How to get the data-id attribute using jQuery
- How to disable/enable submit button after clicked | jQuery
- How to replace innerHTML of a div using jQuery
- How to get the value of a CheckBox with jQuery
- How to wait 'X' seconds with jQuery?
- How to check if an enter key is pressed with jQuery
- How to allow numbers only in a Text Box using jQuery
- How to dynamically create a div in jQuery?