How to replace innerHTML of a div | jQuery
The innerHTML property serves to insert dynamic HTML content within an HTML document. To update or replace this dynamic content, the jQuery .html() function proves invaluable. By invoking this function and supplying the desired new content, developers can efficiently alter the content displayed within an HTML element, ensuring a dynamic and responsive user experience.
The jQuery .html() method serves the purpose of both assigning and retrieving the innerHTML content of selected elements. When utilized to set content, it effectively replaces the existing content within all matched elements. This functionality enables streamlined manipulation of HTML content across multiple elements while maintaining uniformity in the specified changes.

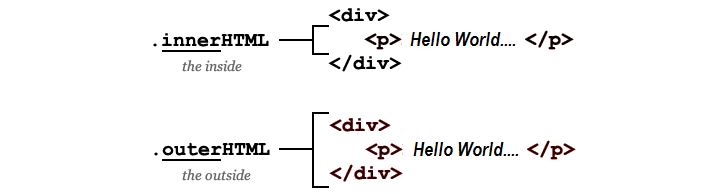
jQuery text()
The jQuery .text() function offers an alternative approach with a slightly different outcome. While both .html() and .text() functions can modify content, the .text() function specifically alters the text value of the designated element while preserving the HTML structure. This distinction allows developers to manipulate textual content without affecting the underlying HTML framework.
Difference between text() and html() method in jQuery
The contrasting behavior between .html() and .text() in jQuery lies in their treatment of content. While .html() interprets the input string as HTML, .text() interprets it as plain text. An important distinction arises when considering the context: .html() lacks availability in XML documents, whereas .text() finds utility in both XML and HTML documents. Moreover, it's noteworthy that .html() exhibits faster performance—twice as fast as .text()—further influencing the selection of the appropriate method based on efficiency.
Change innerHTML content using JavaScript
You can use pure JavaScript to replace innerHTML of a div.
Get innerHTML content using html()
When jQuery .html() method is used to return content, it returns the content of the FIRST matched element.
Conclusion
To replace the innerHTML of a <div> using jQuery, you can utilize the .html() method. By selecting the desired <div> element and applying .html() with the new content, you dynamically update its contents. This approach provides a seamless way to modify and refresh the displayed information within the targeted <div> .
- How to get input textbox value using jQuery
- JQuery Get Selected Dropdown Value on Change Event
- How to submit a form using ajax in jQuery
- How can I get the ID of an element using jQuery
- Open Bootstrap modal window on Button Click Using jQuery
- How to select an element with its name attribute | jQuery
- How to get the data-id attribute using jQuery
- How to disable/enable submit button after clicked | jQuery
- How to get the value of a CheckBox with jQuery
- How to wait 'X' seconds with jQuery?
- How to check if an enter key is pressed with jQuery
- How to allow numbers only in a Text Box using jQuery
- How to dynamically create a div in jQuery?