ValueError: cannot reindex from a duplicate axis
The ValueError "cannot reindex from a duplicate axis" in Pandas occurs when attempting to reindex a DataFrame or Series, but the operation results in duplicate index labels. The reindexing process aims to realign data based on a new set of labels, but if the new labels contain duplicates, it becomes ambiguous how to map the data, leading to this error.
Reindexing with Duplicate Labels
In this example, we have a DataFrame df with duplicate index labels 'x' and 'x'. When we try to reindex the DataFrame with new labels 'x', 'x', and 'z', Pandas raises a ValueError because it cannot determine how to realign the data for the duplicate labels.
Reindexing a Series with Duplicate Labels
In this example, we have a Series series with duplicate index labels 'x' and 'x'. When we try to reindex the Series with new labels 'x', 'x', and 'z', Pandas raises a ValueError due to the presence of duplicate labels.
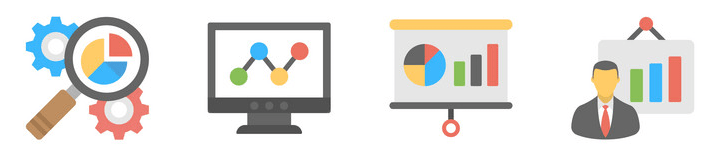
Preserve
If preserving the original DataFrame index values is not a concern, and you prefer unique values for the index, you can achieve this by setting the ignore_index parameter to True.
Overwrite
Alternatively, you can overwrite your current DataFrame index with a new one.
or, use .reset_index:
Remove inplace=True if you want it to return the dataframe.
Prevent
To ensure that your DataFrame does not contain duplicate values in the index, you can set the allows_duplicate_labels flag to False. This prevents the assignment of duplicate values to the index, thereby guaranteeing uniqueness.
Also, consider the following steps:
- Make sure that the new index labels used for reindexing are unique. Avoid using duplicate labels to prevent ambiguity in the alignment of data.
- If you need to deal with duplicate index labels, consider using other Pandas functions like groupby or pivot_table to aggregate or transform the data appropriately.
- If you want to retain duplicate index labels, you can use the duplicated method to identify and handle duplicate rows accordingly before performing the reindexing operation.
Conclusion
Addressing the duplicate index label issue and ensuring a unique set of labels for reindexing, you can avoid the ValueError and successfully realign your data using Pandas reindexing methods.
- ImportError: No module named pandas
- What is SettingWithCopyWarning?
- UnicodeDecodeError while reading CSV file
- How to fix CParserError: Error tokenizing data
- How to fix "Unnamed: 0" column in a pandas DataFrame
- ValueError: cannot convert float NaN to integer
- ValueError: Unknown label type: 'unknown'
- ValueError: Length of values does not match length of index
- ValueError: The truth value of an array with more than..
- Attributeerror: 'dataframe' object has no attribute 'concat'