ValueError: Unknown label type: 'unknown'
The "Unknown label type: 'unknown'" error is typically encountered when working with the Y values in scikit-learn. The error arises due to a discrepancy between the expected input format and the actual data passed. This mismatch could be between Array and DataFrame or 1D list and 2D list, causing scikit-learn to be uncertain about the problem type (regression or classification) and the nature of data in the Y variable. Scikit-learn expects label-like data such as integers or strings, while receiving 'continuous' data (e.g., float numbers), which leads to this error. It is crucial to ensure the correct data format and label type are provided to resolve the issue and effectively utilize scikit-learn for machine learning tasks.
Using 'unknown' Label Type with a Classifier
In this example, we try to fit the SVC model using an 'unknown' label type instead of the correct target labels from the Iris dataset. The model raises a ValueError since it cannot interpret the 'unknown' label as a valid class label.
Using 'unknown' Label Type with Clustering
In this example, we try to fit the KMeans clustering model using a list of 'unknown' label type instead of the actual data samples. The model raises a ValueError because it expects valid data samples for clustering, not labels.
To handle the "Unknown label type: 'unknown'" error, consider the following steps::
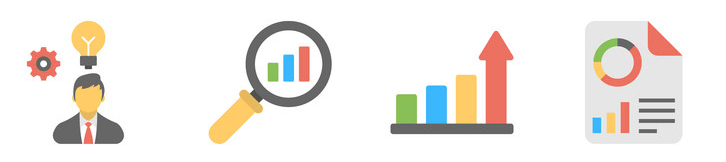
- Double-check the input data and ensure that you are providing the correct and compatible label types expected by the machine learning model.
- Verify that the label data corresponds to the target values or input samples as required by the model.
- Make sure the label type is compatible with the model's requirements. For example, classifiers require discrete class labels, while clustering algorithms require input data samples.
- If you encounter this error while preprocessing data, investigate the data source or the preprocessing steps to ensure the correct label encoding is used.
- It is recommended to discretize or group the Y values into distinct bins or classes, such as 0, 1, 2, 3, using a process called binning. Subsequently, you can use classification modeling techniques on the transformed data. By categorizing the Y values into well-defined classes, you enable the classification algorithms to make accurate predictions based on the assigned bins, facilitating the efficient analysis and interpretation of your data.
- In many cases, the Y values in your dataset are of type 'object', which can cause scikit-learn to have difficulty recognizing the data type. To address this issue, you can explicitly convert the Y values to the integer data type by adding the line y = y.astype('int') before passing the variable into the classifier. This conversion ensures that the Y values are represented as integers, making it easier for scikit-learn to correctly identify the type and enabling smooth execution of the classification algorithms.
- When passing Y values to the rf.fit(X, Y) function in scikit-learn, it expects the Y values to be a 1D list. However, slicing a pandas DataFrame always results in a 2D list. To address this, you need to convert the 2D list provided by the pandas DataFrame to a 1D list as expected by the fit() function. This conversion ensures that the Y values are appropriately represented in a format compatible with scikit-learn's classifier fitting process, allowing for seamless training and accurate model performance.
Conclusion
If your objective is to obtain continuous predictions rather than discrete classes, you should employ regression machine learning methods, such as RandomForestRegressor, to predict the Y values. Regression models are designed to predict continuous numerical values, which aligns with your preference for continuous predictions. By utilizing RandomForestRegressor or similar regression algorithms, you can make accurate predictions on continuous target variables, enabling you to analyze and interpret the data in a more granular manner.
- ImportError: No module named pandas
- What is SettingWithCopyWarning?
- UnicodeDecodeError while reading CSV file
- How to fix CParserError: Error tokenizing data
- ValueError: cannot reindex from a duplicate axis
- How to fix "Unnamed: 0" column in a pandas DataFrame
- ValueError: cannot convert float NaN to integer
- ValueError: Length of values does not match length of index
- ValueError: The truth value of an array with more than..
- Attributeerror: 'dataframe' object has no attribute 'concat'