How to drop "Unnamed: 0" column from DataFrame
To drop the "Unnamed: 0" column from a DataFrame, you can use the drop() method. Here's how you can do it:
The drop() method allows you to specify the column you want to remove using the columns parameter. Setting inplace=True will modify the original DataFrame, while omitting it or setting it to False will create a new DataFrame without the specified column.
"Unnamed: 0"
In certain scenarios, a situation may arise where an "Unnamed: 0" column appears in a pandas DataFrame when reading a CSV file. To address this, a straightforward solution involves treating the "Unnamed: 0" column as the index. To achieve this, you can specify the index_col=[0] argument in the read_csv() function, enabling it to interpret the first column as the DataFrame's index.
index_col=[0]
While you read csv file, if you set index_col=[0] you're explicitly stating to treat the first column as the index.
You can solve this issue by using index_col=0 in you read_csv() function.
index=False
In many instances, the presence of the "Unnamed: 0" index in your DataFrame when using to_csv() is a result of saving the DataFrame with the default index. This can be avoided by using "index=False" as an option while creating the output CSV file, particularly when the DataFrame's index is not required to be included in the saved data.
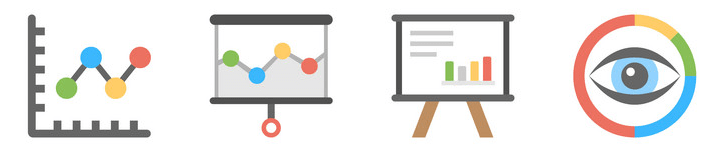
Using regex
You can get ride of all Unnamed columns from your DataFrame by using regex.
Finally, you can simply delete that column using: del df['column_name'] .
Conclusion
To remove the "Unnamed: 0" column from a DataFrame, you can use the drop() method in pandas. Simply specify the column name using the columns parameter, and set inplace=True to modify the original DataFrame or omit it to create a new DataFrame without the specified column.
- ImportError: No module named pandas
- What is SettingWithCopyWarning?
- UnicodeDecodeError while reading CSV file
- How to fix CParserError: Error tokenizing data
- ValueError: cannot reindex from a duplicate axis
- ValueError: cannot convert float NaN to integer
- ValueError: Unknown label type: 'unknown'
- ValueError: Length of values does not match length of index
- ValueError: The truth value of an array with more than..
- Attributeerror: 'dataframe' object has no attribute 'concat'