The truth value of an array with more than one..
The ValueError "The truth value of an array with more than one element is ambiguous. use a.any() or a.all()" occurs in Python when trying to evaluate the truth value of an array that has more than one element. This ambiguity arises because the condition being evaluated could be true for some elements and false for others, making it uncertain whether the entire array should be considered true or false.
Python boolean
In Python, boolean values are limited to either True or False. Nonetheless, when dealing with NumPy arrays, it is feasible to encounter arrays comprising multiple elements, where each element may assume a True or False value. Consequently, in such scenarios, the determination of whether the entire array should be considered True or False becomes ambiguous due to the presence of multiple elements with potentially differing truth values.
How to Fix?
To resolve this, you can use the a.any() function to check if any element in the array is true or a.all() function to check if all elements in the array are true.
In this example, we have an array arr with multiple elements (3 elements). When we attempt to evaluate the truth value of the array in the condition if arr, Python raises a ValueError because it is unclear whether the entire array should be considered true or false.
To handle this error, consider the following approaches:
If you want to check if any element in the array is true, use if arr.any().
Numpy any() Vs all()
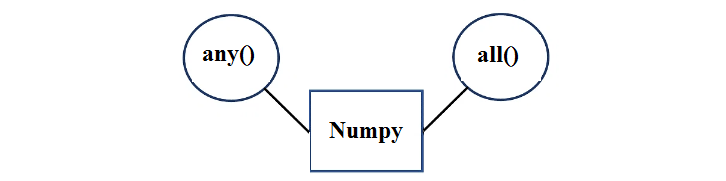
NumPy's any() and all() are useful methods used to assess whether any or all elements of a given NumPy array fulfill a specified condition. The any() method returns a boolean value of True if at least one element meets the condition, while the all() method returns True only if all elements satisfy the condition. These methods are valuable tools for performing conditional checks and obtaining concise results when working with NumPy arrays.
Numpy any()
The any() method returns True if at least one element of the array satisfies the given condition.
In the above example, the any() method is used to check whether any element in the array arr is greater than zero. Since there are elements that are greater than zero, any() returns True.
Numpy all()
On the other hand, the all() method returns True only if all the elements in the array satisfy the given condition. For example:
In the above example, the all() method is used to check whether all elements in the array arr are greater than zero. Since there are elements that are not greater than zero, all() returns False.
Both any() and all() can also be used with multi-dimensional arrays. In such cases, you can specify the axis along which to apply the method.
In the above example, the any() method is used to check whether any element in each column of the two-dimensional array arr is greater than 4. The axis parameter is set to 0 to indicate that the method should be applied to each column. The output is an array with one value for each column, indicating whether any element in that column greater than 4.
NumPy | Python library
NumPy is a powerful Python library that plays a crucial role in scientific computing and data analysis. It offers robust support for handling large, multi-dimensional arrays and matrices, making it highly efficient for numerical computations and data manipulation. NumPy's extensive collection of mathematical functions empowers users to perform complex operations on arrays with ease. Due to its versatility and performance, NumPy finds widespread applications across various domains, including physics, engineering, finance, and machine learning. As a fundamental tool for data processing in Python, NumPy proves indispensable for anyone dealing with data-related tasks and analyses.
Conclusion
The ValueError "The truth value of an array with more than one element is ambiguous. use a.any() or a.all()" arises when attempting to evaluate the truth value of a NumPy array with multiple elements, which can have varying True or False values. To resolve this ambiguity, one can use a.any() to check if at least one element is True, or a.all() to verify if all elements are True in the array.
- ImportError: No module named pandas
- What is SettingWithCopyWarning?
- UnicodeDecodeError while reading CSV file
- How to fix CParserError: Error tokenizing data
- ValueError: cannot reindex from a duplicate axis
- How to fix "Unnamed: 0" column in a pandas DataFrame
- ValueError: cannot convert float NaN to integer
- ValueError: Unknown label type: 'unknown'
- ValueError: Length of values does not match length of index
- Attributeerror: 'dataframe' object has no attribute 'concat'