Convert Python dict to Pandas Dataframe
Converting a Python dictionary to a Pandas DataFrame is a fundamental data manipulation task, as it enables data professionals to use Pandas' powerful data structures and functionalities for analysis and visualization. The process involves transforming the key-value pairs of a dictionary into columns and rows of a DataFrame, effectively organizing the data in a tabular format.
Pandas provides a convenient function called pd.DataFrame() that can be used for this conversion. Let's explore how to convert a Python dictionary to a Pandas DataFrame with examples:
Simple dictionary with lists as values
Suppose we have a Python dictionary containing information about students:
In this example, the Python dictionary data is converted into a Pandas DataFrame df. Each key in the dictionary becomes a column, and the corresponding lists as values populate the rows of the DataFrame.
Dictionary with nested dictionaries as values
Suppose we have a Python dictionary containing information about students with additional details:
In this example, the Python dictionary data contains a nested dictionary with details for each student. When converted to a Pandas DataFrame, the 'Details' key becomes a column containing dictionaries as values.
Dictionary Keys and Values as DataFrame rows
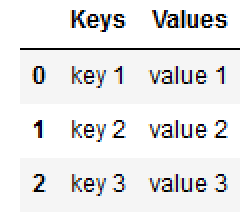
Dictionary Keys and Values as DataFrame rows
Keys to DataFrame Column and Values to DataFrame Rows
Keys to DataFrame Column and Values to DataFrame Rows
Keys to DataFrame Column and Values to DataFrame Rows
Keys to DataFrame Rows and Values to DataFrame columns
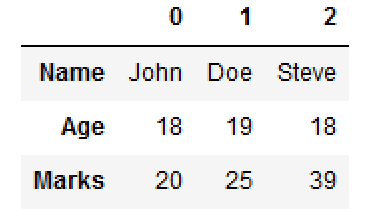
Pandas automatically aligns data in a tabular format while converting a dictionary to a DataFrame, and it also handles missing values appropriately.
Conclusion
In conclusion, the process of converting a Python dictionary to a Pandas DataFrame is a seamless and versatile operation that enhances the data exploration and analysis capabilities of Python. By utilizing the power of Pandas, data professionals can manipulate, visualize, and derive insights from data with elegance and precision. The DataFrame structure, fortified by the essence of Python dictionaries, stands as a cornerstone in the data science landscape, elevating Python's position as a dominant force in data-driven knowledge.
- Pandas DataFrame: GroupBy Examples
- Pandas DataFrame Aggregation and Grouping
- How to Sort Pandas DataFrame
- Pandas DataFrame: query() function
- Finding and removing duplicate rows in Pandas DataFrame
- How to Replace NaN Values With Zeros in Pandas DataFrame
- How to read CSV File using Pandas DataFrame.read_csv()
- How to Convert Pandas DataFrame to NumPy Array
- How to shuffle a DataFrame rows
- Import multiple csv files into one pandas DataFrame
- Create new column in DataFrame based on the existing columns
- New Pandas dataframe column based on if-else condition
- Rename Pandas columns/index names (labels)
- Check for NaN Values : Pandas DataFrame