Convert Pandas DataFrame to NumPy Array
The seamless interoperability between Pandas and NumPy empowers data analysts and scientists to embark on a transformative journey of data manipulation and analysis. One key facet of this synergy lies in the capability to convert a Pandas DataFrame, a versatile tabular data structure, into a NumPy array, a fundamental and efficient data container in Python.
Convert pandas dataframe to NumPy array
- Usng to_numpy()
- Using to_records()
- Using asarray()
Lets craete a DataFrame..
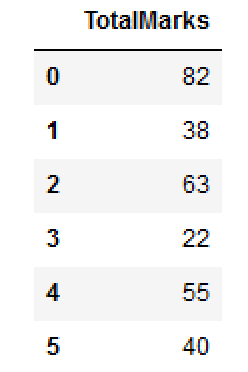
The process of converting a Pandas DataFrame to a NumPy array is enabled by the values attribute, which unfolds the DataFrame's contents into a multi-dimensional NumPy array representation. This attribute unlocks a gateway to unlock a treasure trove of data manipulation possibilities, as NumPy arrays offer a streamlined and optimized foundation for numerical computations and mathematical operations.
Convert pandas dataframe to NumPy array usng to_numpy()
NumPy array dtype
In the above output you can see the dtype=int64 . You can also provide dtype=int64 as parameters.
Once the conversion to a NumPy array is accomplished, data professionals can use the vast ecosystem of NumPy's array-based functionalities. From applying statistical functions and linear algebra operations to employing advanced indexing and slicing techniques, the NumPy array unfolds as a canvas for data exploration and modeling endeavors.
Convert pandas dataframe to NumPy array usng to_records()
Note that this is a recarray rather than an numpy.ndarray. You could move the result in to regular numpy array by calling its constructor as np.array(df.to_records()) .
The transition from a Pandas DataFrame to a NumPy array is a prudent choice when dealing with large datasets, as NumPy's underlying C implementation grants exceptional computational efficiency and performance. This seamless migration ensures that data-intensive tasks, such as machine learning algorithms or numerical simulations, are executed with precision and speed.
Convert pandas dataframe to NumPy array usng asarray()
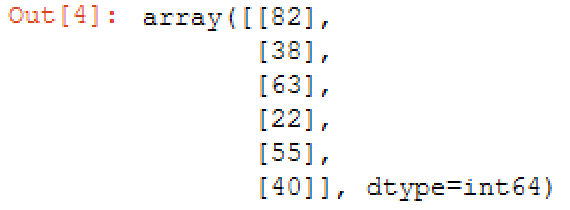
df.values and df.as_matrix()
You can use df.values and df.as_matrix() to convert dataframe to NumPy array. But these two methods are depreciated. If you visit the v0.24 docs for .values, you will see a big red warning that says:
Conclusion
The ability to convert a Pandas DataFrame to a NumPy array represents a foundational step towards unleashing the true potential of data analysis and scientific computing in Python. This harmonious integration of data structures develops a holistic approach to data-driven solutions, as data professionals can seamlessly traverse between the scope of tabular data manipulation and array-based numerical computations with grace and fluency. By utilizing this interoperability, data analysts and scientists can unlock deeper insights, make data-informed decisions, and drive transformative outcomes with finesse and accuracy.
- Pandas DataFrame: GroupBy Examples
- Pandas DataFrame Aggregation and Grouping
- How to Sort Pandas DataFrame
- Pandas DataFrame: query() function
- Finding and removing duplicate rows in Pandas DataFrame
- How to Replace NaN Values With Zeros in Pandas DataFrame
- How to read CSV File using Pandas DataFrame.read_csv()
- How to shuffle a DataFrame rows
- Import multiple csv files into one pandas DataFrame
- Create new column in DataFrame based on the existing columns
- New Pandas dataframe column based on if-else condition
- How to Convert a Dictionary to Pandas DataFrame
- Rename Pandas columns/index names (labels)
- Check for NaN Values : Pandas DataFrame