Rename DataFrame columns/index names
Renaming DataFrame columns and index names is a common operation that allows data professionals to customize the DataFrame's structure to their requirements, improve readability, and provide more informative labels. Pandas provides several methods to achieve this, including rename(), columns, index, and assigning new values directly. You can simply rename DataFrame column names using DataFrame.rename() .
Lets create a DataFrame..
Rename DataFrame column using DataFrame.rename()
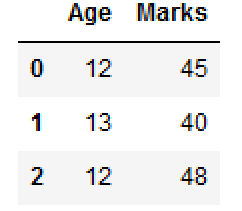
Rename DataFrame index using DataFrame.rename()
Reassign DataFrame column/index names using set_axis()
Changing DataFrame Index label using DataFrame.set_axis()
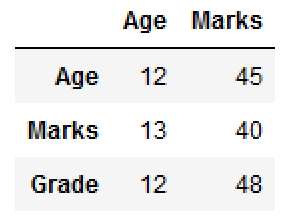
Conclusion
Renaming DataFrame columns and index names in Pandas is a straightforward operation that offers flexibility in customizing the DataFrame's structure. Whether using the rename() method or directly assigning new values to .columns and .index attributes, data professionals can tailor the DataFrame's labels to enhance data clarity and analytical insights. This ability to rename columns and index names adds to the versatility of Pandas in providing intuitive data manipulation and visualization for effective data-driven solutions.
- Pandas DataFrame: GroupBy Examples
- Pandas DataFrame Aggregation and Grouping
- How to Sort Pandas DataFrame
- Pandas DataFrame: query() function
- Finding and removing duplicate rows in Pandas DataFrame
- How to Replace NaN Values With Zeros in Pandas DataFrame
- How to read CSV File using Pandas DataFrame.read_csv()
- How to Convert Pandas DataFrame to NumPy Array
- How to shuffle a DataFrame rows
- Import multiple csv files into one pandas DataFrame
- Create new column in DataFrame based on the existing columns
- New Pandas dataframe column based on if-else condition
- How to Convert a Dictionary to Pandas DataFrame
- Check for NaN Values : Pandas DataFrame