Java HashMap
A HashMap is a useful data structure when you need to associate keys with values, such as when you need to maintain a mapping of names to employee IDs, or when you need to associate product codes with product descriptions. The HashMap class provides methods for inserting and retrieving key-value pairs, as well as methods for modifying and removing elements.
Here's a simple example to demonstrate the basic usage of the java.util.HashMap class:
This example creates a HashMap that maps keys and values. The put method is used to add key-value pairs to the map. The get method is used to retrieve a value using a key. The containsKey method is used to check if a key exists in the map. The remove method is used to remove a key-value pair using a key. Finally, the keySet method is used to get a set of the keys in the map, and a for loop is used to iterate over the key-value pairs and print them.
What is Java hashmap
The java.util.HashMap is a class in Java that implements the Map interface. It stores key-value pairs in a hash table, allowing for constant-time (O(1)) access to individual elements based on their keys. The HashMap class uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. This allows for efficient insertion and retrieval of elements, and also helps to ensure that the HashMap remains balanced as elements are added and removed.
Important Features of HashMap
Some of the important features of the java.util.HashMap class include:
- Key-Value Mapping: The HashMap stores key-value pairs, where each key is associated with a single value. This allows you to retrieve the value associated with a given key in constant time (O(1)).
- Hashing: The HashMap uses a hash function to compute an index into an array of buckets or slots, where the key-value pairs are stored. This allows for efficient insertion and retrieval of elements, and helps to ensure that the HashMap remains balanced as elements are added and removed.
- Dynamic Size: The HashMap is dynamically resizable, which means that it can grow or shrink as elements are added or removed. This makes it a useful data structure for applications that need to store a large number of elements that may change over time.
- Null Keys and Values: The HashMap allows for null keys and values, which can be useful in certain situations.
- Concurrent Access: The HashMap is not synchronized, which means that multiple threads can access it simultaneously. This can lead to data corruption if multiple threads modify the HashMap simultaneously. To overcome this, you can use the java.util.Collections.synchronizedMap(Map
m) method to obtain a synchronized (thread-safe) map. - Iteration: The HashMap provides methods for iterating over the key-value pairs, such as the keySet(), values(), and entrySet() methods.
How Java hashmap works?
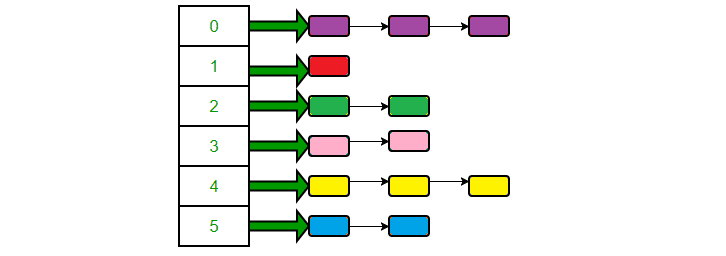
The Java HashMap works by using a hash function to compute an index into an array of buckets or slots, where the key-value pairs are stored. The hash function takes the key as input and returns an integer value, which is used as the index into the array.
When a key-value pair is added to the HashMap, the hash function is used to compute the index for the key. If the index is already occupied, the HashMap checks the keys stored at that index to determine if the key already exists. If it does, the old value is replaced with the new HashMap value. If the key does not already exist, the key-value pair is added to the HashMap at that index.
When a value is retrieved from the HashMap, the hash function is used to compute the index for the key. The HashMap then checks the keys stored at that index to determine if the key exists. If it does, the associated value is returned. If the key does not exist in HashMap, null is returned.
The HashMap also uses a technique called rehashing to ensure that the keys are evenly distributed across the buckets. Rehashing occurs when the HashMap reaches a certain threshold of utilization, at which point the size of the HashMap is increased and the keys are rehashed to their new indices. This helps to ensure that the HashMap remains balanced and efficient as elements are added and removed.
Overall, the HashMap provides constant-time (O(1)) access to individual elements based on their keys, making it a fast and efficient data structure for storing key-value pairs in Java.
Internal Structure of HashMap
Java HashMap is implemented as an array of buckets, where each bucket is a linked list of key-value pairs. Each key-value pair is represented as a Map.Entry object, which stores the key and value, as well as a reference to the next entry in the same bucket. When you add a new entry to the HashMap, it uses the hash code of the key to determine the index of the bucket where the entry should be placed. If the bucket already contains one or more entries, the new entry is appended to the end of the linked list. To look up a value by its key, the HashMap calculates the hash code of the key and then searches the linked list at the corresponding bucket for the desired entry.
Java Hashmap Examples:
Following are some examples of using a HashMap in Java:
Creating a HashMap
In the above example, the HashMap is created with String keys and Integer values.
Add elements in Hashmap
Elements can be added to a java.util.HashMap using the put method:
The put method in HashMap takes two arguments: a key and a value. The key is used to look up the value in the map, and the value is the value that is associated with the key. In this example, the keys are strings (the names), and the values are integers (the ages). You can add as many key-value pairs as you want to the map, and you can look up the values using the keys later.
Remove elements from HashMap
The following example that demonstrates how to remove elements from a HashMap using the remove method:
In the above example, the remove() method is used to remove a key-value pair from the map. The method takes a single argument, which is the key of the key-value pair to be removed. In this example, the key is "Two". The remove method returns the value that was associated with the key, or null if the key was not found in the map. In this example, the map is printed before and after removing an element, so you can see the effect of the remove method.
How to update a hashmap value by using key?
Following example that demonstrates how to replace elements in a HashMap using the put method:
In this example, the put method is used to replace an element in the map. The HashMap method takes two arguments: a key and a value. The key is used to look up the value in the map, and the value is the value that is associated with the key. In this example, the key is "Two", and the value is 22. If the key already exists in the map, the put method will replace the existing value with the new value. In this example, the map is printed before and after replacing an element, so you can see the effect of the put method.
Search a key in HashMap
To search for a key in a java.util.HashMap, you can use the containsKey method, like this:
In this example, the containsKey method is used to search for a key in the map. The method takes a single argument, which is the key to be searched for. In this example, the key is "Two". The containsKey method returns true if the key is found in the map, and false otherwise. In this example, the result of the search is printed to the console.
How to get Key from Value in HashMap?
To get the key associated with a value in a java.util.HashMap, you can loop over the entry set of the map and check each value.
In this example, the getKey method is used to get the key associated with a value in the map. The method takes two arguments: the map and the value. The method loops over the entry set of the map and checks each value. If a value is found that matches the value being searched for, the method returns the associated key. In this example, the key is associated with the value 2 and is printed to the console.
Iterate over the elements in Hashmap
There are several ways to iterate over the elements of a java.util.HashMap:
Using the keySet method:
Using the entrySet method:
In both examples, the elements of the map are printed to the console. Using the keySet method, the keys are looped over and the associated values are retrieved using the get method. Using the entrySet method, the entries of the map are looped over directly and the keys and values are retrieved using the getKey and getValue methods, respectively.
Get Size of Java HashMap?
To get the size of a HashMap, you can use the size method:
In this example, the size of the map is calculated using the size method and printed to the console. The output will be: Size of the HashMap: 3.
Java HashMap Methods (Summary):
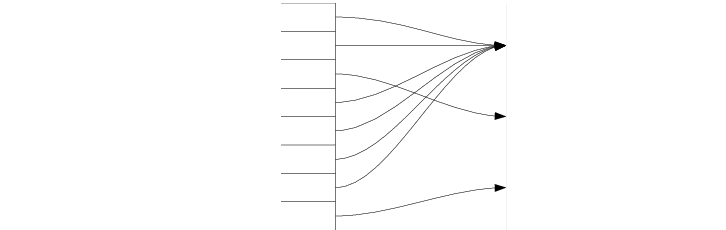
The HashMap class in Java provides several methods to manipulate a HashMap. Some of the most commonly used HashMap methods are:
- put(K key, V value): Adds an element to the HashMap with the specified key and value.
- get(Object key): Returns the value associated with the specified key in the HashMap.
- remove(Object key): Removes the key-value pair with the specified key from the HashMap.
- containsKey(Object key): Returns true if the HashMap contains the specified key, and false otherwise.
- containsValue(Object value): Returns true if the HashMap contains the specified value, and false otherwise.
- size(): Returns the number of key-value pairs in the map.
- isEmpty(): Returns true if the HashMap is empty, and false otherwise.
- clear(): Removes all the key-value pairs from the HashMap.
- keySet(): Returns a Set view of all the keys in the map.
- values(): Returns a Collection view of all the values in the HashMap.
- entrySet(): Returns a Set view of all the key-value pairs in the HashMap.