Hashtable
Hashtable class is a data structure that implements the Map interface and provides an efficient way to store key-value pairs. It is similar to the HashMap class, but it is synchronized, meaning that it is thread-safe and can be used in multi-threaded environments without the need for external synchronization.
Hashtable Properties:
- Keys and values in a Hashtable can be of any non-null object type.
- Hashtable does not allow duplicate keys. If you attempt to add a duplicate key, the existing value will be replaced.
- Both keys and values can be null. However, a null key is not allowed since Hashtable uses the key's hash code for indexing.
- The order of elements in a Hashtable is not guaranteed. If you need an ordered collection, you can use the LinkedHashMap class instead.
Creating a Hashtable:
In this example, we create a Hashtable that stores key-value pairs, where the keys are of type String and the values are of type Integer.
Adding Elements to the Hashtable:
We can add elements to the Hashtable using the put() method. Each element consists of a key-value pair, where the key uniquely identifies the value. In this case, we associate the keys "apple", "banana", and "orange" with their respective values.
Retrieving Values from the Hashtable:
We can retrieve values from the Hashtable using the get() method by specifying the key. In this example, we retrieve the value associated with the key "apple" and store it in the appleCount variable.
Checking if a Key Exists in the Hashtable:
We can check if a key exists in the Hashtable using the containsKey() method. It returns true if the specified key exists in the Hashtable; otherwise, it returns false.
Removing Elements from the Hashtable:
We can remove elements from the Hashtable using the remove() method by specifying the key. In this case, we remove the key-value pair associated with the key "orange".
Iterating over the Hashtable:
We can iterate over the elements of the Hashtable using a for-each loop and the entrySet() method. This allows us to access both the keys and values of each element in the Hashtable.
Some features and characteristics of the Hashtable class:
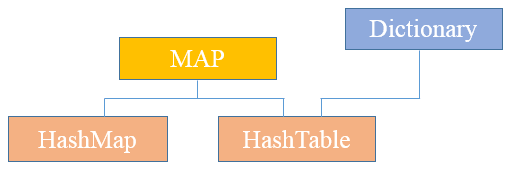
- Key-Value Pairs: Hashtable stores data in the form of key-value pairs, where each key is unique within the Hashtable.
- Synchronization: Hashtable is synchronized, which means it is thread-safe. Multiple threads can safely access and modify a Hashtable concurrently without causing data corruption.
- Null Values: Both keys and values in a Hashtable can be null. However, a null key is not allowed, as Hashtable uses the key's hash code for indexing and null does not have a hash code.
- Performance: Hashtable offers constant-time performance for basic operations like get, put, and remove. However, the actual performance can vary depending on factors such as the size of the Hashtable, the hash function, and the number of collisions.
- Iteration: You can iterate over the elements of a Hashtable using iterators or the Enumeration interface, which provides a legacy mechanism for iterating over Hashtable elements.
- Resizing: When the number of elements in a Hashtable exceeds a certain threshold, the Hashtable automatically resizes itself to accommodate more elements. This resizing involves rehashing all the existing elements into a larger hash table, which can be a computationally expensive operation.
- Ordering: Unlike some other data structures, Hashtable does not guarantee any specific order for its elements. The order in which elements are stored and retrieved may not be the same.
Conclusion
HashMap provides similar functionality and performance advantages while not being synchronized. However, if you require thread-safe operations or need to work with legacy code that specifically uses Hashtable, it can still be a viable option.