Java Record Class with Examples
Java Record is a new feature in Java 14 (released in March 2020) that provides a compact syntax for declaring classes that act as transparent holders for shallowly immutable data. It is intended to act as a replacement for data classes or POJOs (Plain Old Java Objects) that are commonly used to hold data with little or no behavior.
Records were designed to address some of the limitations of POJOs, such as verbose code, lack of type safety, and limited ability to automatically generate useful methods such as toString(), hashCode(), and equals().
Java Record declaration
Here's an example of a simple record declaration in Java:
With this declaration, the Java compiler automatically generates the following methods for the record:
- A constructor that takes arguments for each component of the record.
- Accessors (getters) for each component of the record.
- toString(), hashCode(), and equals() methods based on the components of the record.
Java Record Constructor
A Java Record Constructor is a special constructor generated by the Java compiler for a Record class. The constructor is generated based on the components (fields) of the record, and is used to initialize the values of those components when a new instance of the record is created.
The constructor is generated automatically by the Java compiler, so you don't need to write it yourself. The constructor takes as arguments one value for each component of the record, and uses those values to initialize the corresponding fields.
Here's an example of a Record class and its generated constructor:
The Java compiler automatically generates the following constructor for this record:
Note that the generated constructor is public, so it can be called from anywhere. You can also add additional constructors or methods to the record if desired, but the generated constructor must always be present.
Java Record | Example
This declares a new record class Point with two components (fields), x and y, both of type int. With this declaration, the Java compiler automatically generates the following methods for the record:
Note how you can create a new instance of the Point record using the constructor generated by the Java compiler, and how you can access the components (fields) of the record using the automatically generated accessors. you can also use the automatically generated toString() method to print a human-readable representation of the record, and the equals() method to compare two instances of the record for equality.
Restrictions on java Records
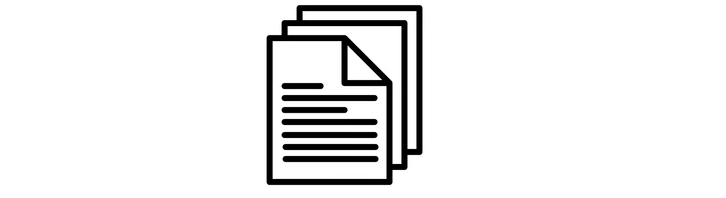
Java Records have some restrictions that you should be aware of when using them:
- Records can only have final instance variables: The components (fields) of a record must be declared as final. This means that the values of the components cannot be changed after the record is created.
- Records cannot have instance initializers: Instance initializers are blocks of code that are executed when an instance of a class is created. Records do not support instance initializers, so you cannot use them to initialize the components of a record.
- Records cannot extend other classes: Records are a new kind of type in Java, and they cannot extend other classes. This means that a record can only inherit the methods and fields defined in java.lang.Record.
- Records cannot have instance methods with the same signature as their components: The Java compiler generates accessors (getters) for each component of a record. You cannot declare an instance method with the same name as a component, as this would result in a method signature conflict with the generated accessor.
- Records cannot be abstract: Records must have a complete implementation, and cannot be declared as abstract.
These restrictions are designed to enforce the idea that records are simple data structures that are meant to hold data, and to prevent records from being used in ways that might compromise their type safety or their performance. By following these restrictions, you can ensure that your records are used correctly and efficiently, and that they provide the benefits that they were designed to deliver.
Why do you need Java Records?
Java Records were introduced in Java 14 as a new feature to provide a more concise and type-safe way to model simple data structures. Here are some of the reasons why you might want to use records in your Java code:
- Concise syntax: Records provide a compact syntax for declaring classes that hold data. This makes your code more readable and less verbose compared to traditional Java classes, especially when you're dealing with simple data structures.
- Improved type safety: Records provide built-in type safety, since the components of a record are declared as fields with specific types. This helps prevent bugs and makes your code more maintainable.
- Automated methods: Records automatically generate useful methods such as toString(), hashCode(), and equals() based on the components of the record. This saves you from having to write these methods yourself, and ensures that they're correct and consistent with your code.
- Better support for value-based programming: Records are designed to be used as value types, meaning that instances of a record are meant to be treated as if they were primitive values. This makes it easier to use them as keys in maps, for example, or as elements in collections.
- Easier migration from data classes: If you're coming from a language that supports data classes (such as Kotlin), records provide a similar way to model simple data structures in Java. This makes it easier to migrate your code to Java, and to work with libraries that use data classes.
In general, Java Records are a great choice when you need to model simple data structures in your code. They provide a more concise and type-safe way to do so compared to traditional Java classes, and they make it easier to write correct and maintainable code.