Java HashSet Class
HashSet class extends AbstractSet and implements the set interface . A set is a collection that contains no duplicate elements, and whose elements are in no particular order. In HashSet , hash table is used for storage. A hash table stores information by using a mechanism called hashing. In simple, hashing is a way to assigning a unique code for any variable/object after applying any formula/algorithm on its properties. Hash function should return the same hash code each and every time, when function is applied on same or equal objects.
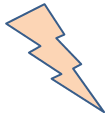
Creates a hash set and initializes both the capacity and fill ratio of the hash set from its arguments. The fill ratio must be between 0.0 and 1.0. When the number of elements is greater than the capacity, the capacity of the hash set is multiplied with the fill ratio and the hash set is expanded.
A HashSet holds a set of objects, but in a way that it allows you to easily and quickly determine whether an object is already in the set or not. It does so by internally managing an array and storing the object using an index which is calculated from the hashcode of the object. There are different implementations of Sets. Some make insertion and lookup operations super fast by hashing elements. However that means that the order in which the elements were added is lost. Other implementations preserve the added order at the cost of slower running times.
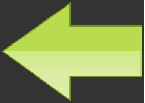
HashSet makes no guarantees as to the iteration order of the set; in particular, it does not guarantee that the order will remain constant over time. In general, Sets implemented using hashing have no well-defined order. Because there is a hash value calculated for each object and this hash value determines the array index of the particular object in the container. So the order of inserted elements are naturally not preserved. If you want to keep insertion order by using HashSet than consider using LinkedHashSet.
The following Java program illustrates several of the methods supported by this HashSet collection Framework