Python any() Vs all()
In Python, the any() and all() functions are used to determine if the elements in an iterable object meet a certain condition. The main difference between the two functions is the logical operation they use to combine the conditions.
- any(): Returns True if at least one element in the iterable object is true. Otherwise, returns False.
- all(): Returns True if all elements in the iterable object are true. Otherwise, returns False.
Following is an example that demonstrates the difference between any() and all():
In the above example, there is a list of numbers. Use a generator expression to check if any of the numbers in the list are odd (num % 2 == 1) using any(). Since the number 9 is odd, any() returns True.
Also use a generator expression to check if all the numbers in the list are even (num % 2 == 0) using all(). Since the number 9 is odd, all() returns False.
When to use Python any() Vs all()?
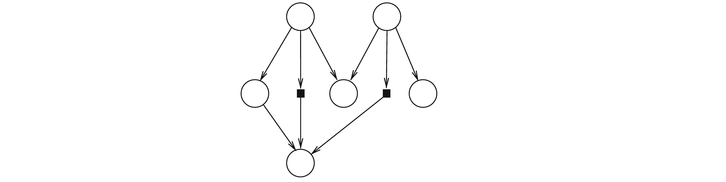
You can use Python's any() and all() functions when you want to check whether the elements in an iterable object meet a certain condition.
- Use any(): when you want to check if at least one element in an iterable object meets a condition. For example, you might use any() to check if a list of numbers contains any negative numbers.
- Use all(): when you want to check if all elements in an iterable object meet a condition. For example, you might use all() to check if all the elements in a list of boolean values are True.
Both any() and all() can be used with any iterable object, including lists, tuples, sets, and generators. They are also both short-circuiting, which means they will stop iterating over the iterable as soon as they can determine the final result.
It's worth noting that any() and all() can also be combined with other logical operators, such as not and or, to create more complex conditions. For example, you might use any() with the not operator to check if no elements in an iterable meet a certain condition.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python