Python any() Function with Examples
In Python, the any() function is a built-in function that takes an iterable object (such as a list, tuple, or set) as an argument and returns True if at least one element in the iterable object evaluates to True, and False otherwise.
Following are some possible ways to use any() in Python:
Python any() function with Strings
When used with strings, any() will return True if any character in the string evaluates to True (meaning not an empty string, None, or 0).
Following is an example of using any() with a string:
In the above example, first check if any character in the string s is a digit by using the isdigit() method. Since there are no digits in the string, any() returns False.
Next, check if any character in the string s is lowercase by using the islower() method. Since there are lowercase characters in the string ("hello world"), any() returns True.
Using Python any() with a function
You can use Python any() with a function that returns a boolean value to check if any element in an iterable satisfies a condition.
Following is an example of how to use any() with a function:
In the above example, define a function is_even that takes a number as input and returns True if the number is even. Also create a list of numbers called numbers.
Then use any() with a generator expression that applies the is_even function to each element in numbers. The generator expression returns a Boolean value for each element in numbers, indicating whether the element is even or not. The any() function then returns True if at least one of the Boolean values is True, indicating that at least one element in numbers is even.
In this case, since 6 is even, the output will be "At least one number is even."
To Combine Multiple Conditions with Logical OR
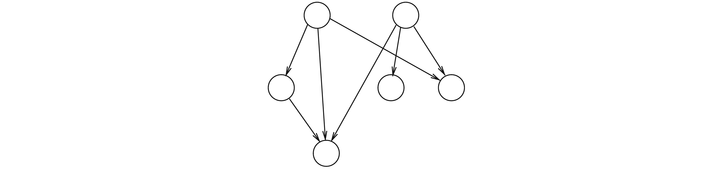
Following is an example that combines multiple conditions using any() with logical OR:
In the above example, there are three variables x, y, and z. Use any() to check if any of the following conditions are true: x < y, x < z, or y < z. Since the third condition y < z is true (5 < 7) , any() returns True.
Note that here pass the conditions as a list to any(). This is because any() expects an iterable as its argument, and a list is a common iterable type in Python. You could also pass a tuple, set, or other iterable type to any().
Using a list:
In the above example, any() checks if at least one element in the list my_list is True, which is the case, so it returns True.
Using a tuple:
In the above example, any() checks if at least one element in the tuple my_tuple is True, but all the elements evaluate to False, so it returns False.
Using a set:
In the above example, any() checks if at least one element in the set my_set is True, which is the case for the integers 1 and 2, so it returns True.
Using a generator expression:
In the above example, any() checks if at least one element generated by the expression (i > 0) for each element i in the list [1, 2, -3] is True. Since the first two elements are greater than zero, any() returns True.
Using Dictionaries
When used with dictionaries, the any() function in Python will check if any of the keys or values in the dictionary evaluate to True.
Following is an example of using any() with a dictionary:
In the above example, there is a dictionary my_Dict with three key-value pairs. The value of the "name" key is a non-empty string, the value of the "age" key is a non-zero integer, and the value of the "email" key is an empty string.
The first call to any() checks if any of the values in the dictionary are true. Since the value of the "name" key is a non-empty string, any() returns True.
The second call to any() checks if any of the keys in the dictionary are true. Since all the keys are non-empty strings, any() returns True.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python