Modulenotfounderror: no module named 'apt_pkg'
The ModuleNotFoundError: No module named 'apt_pkg' error occurs when Python is unable to find and import the apt_pkg module. This module is typically used in Debian-based Linux distributions to interact with the Advanced Package Tool (APT) for managing software packages.
There are several possible reasons why this error might occur:
Module may not be installed on your system
You can install it by running the following command in your terminal:
Module may not be installed in the correct location
You can check the location of the module by running the following command in your Python console:
This will print the file path of the apt_pkg module. If the module is not located in a directory that Python searches by default, you can add the directory to the PYTHONPATH environment variable.
May not have permission to access the apt_pkg module
This can occur if the module is installed in a system directory that requires root access. You can try running your Python code with elevated privileges using the sudo command.
Following is an example of how this error might occur:
To resolve this error, you should first try installing the python-apt package using the apt-get command, as mentioned above. If that doesn't work, you can try checking the location of the apt_pkg module and adding the directory to the PYTHONPATH environment variable.
Add directory | PYTHONPATH environment variable
You can add a directory to the PYTHONPATH environment variable in Linux by following these steps:
Open the terminal and enter the following command to determine the current value of the PYTHONPATH environment variable:
If the variable is not set, the output will be blank.
Determine the path of the directory you want to add to the PYTHONPATH variable.
Set the PYTHONPATH environment variable to include the new directory by running the following command:
Replace /path/to/new/directory with the actual path to the directory you want to add.
To verify that the directory has been added to the PYTHONPATH environment variable, run the following command:
The output should include the path to the new directory.
Note that setting the PYTHONPATH environment variable in this way will only affect the current terminal session. To make the change permanent, you can add the export command to your shell's startup script, such as ~/.bashrc or ~/.bash_profile.
apt_pkg module Python
Python module apt_pkg is used in Debian-based Linux distributions to interact with the Advanced Package Tool (APT) for managing software packages. APT is a command-line tool that is used to install, update, and remove software packages on a Linux system. It works by connecting to a software repository, downloading package information, and resolving dependencies.
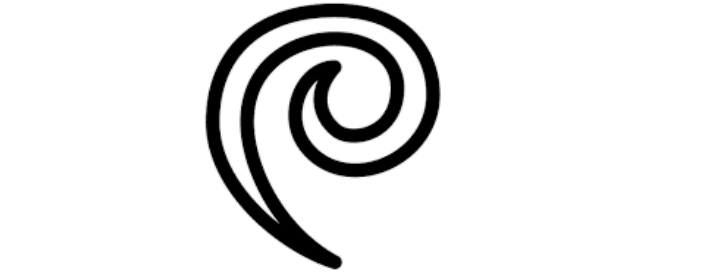
The apt_pkg module provides a Python interface to the APT system, allowing developers to write scripts that can perform package management tasks programmatically. Some of the tasks that can be performed using apt_pkg include:
- Retrieving information about installed packages
- Installing and removing packages
- Upgrading packages to newer versions
- Managing package repositories
- Searching for packages in the repository
The apt_pkg module is typically used in Python scripts that automate software management tasks on a Linux system. For example, a script might use apt_pkg to install a list of required packages for a particular application, or to check for updates to installed packages.
Following is an example of how apt_pkg might be used in a Python script:
In the above code, the init_system() function is used to initialize the APT system, and the Cache() class is used to get a list of all installed packages. The Package() class is used to create a new package object, which is marked for installation using the mark_install() method. Finally, the changes to the package cache are committed using the commit() method.
Note that apt_pkg is only available on Debian-based Linux distributions that use APT as their package management system. Other Linux distributions may use different package management tools, such as yum or dnf, which have their own corresponding Python modules.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Convert List to Array Python