Custom Exceptions in Python
Exceptions in Python are events that occur during the execution of a program that disrupt the normal flow of the program's instructions. When an exception occurs, the program will stop executing and instead raise an error message that describes the nature of the problem.
Python Custom Exceptions
User-Defined Exceptions in Python are custom exceptions that are created by the programmer to handle specific errors that may occur in their code. These exceptions are based on the built-in Exception class in Python and can be raised using the raise statement.By defining their own exceptions, programmers can create more meaningful error messages and provide better guidance to users on how to resolve the issues that have occurred. User-defined exceptions can also be used to control the flow of the program and handle specific error scenarios in a more efficient way.
Custom Exception | Example
Following is an example of how to define and use a custom exception in Python:
In the above code, define a custom exception class NegativeNumberException, which is raised when a negative number is encountered. This exception class inherits from the base Exception class, and provides a custom message in the __str__ method to indicate the negative number that was encountered.
Then define a function square_root(x), which calculates the square root of a number x. If the input is negative, it raises a NegativeNumberException with the value of x.
In the try-except block, call the square_root function with a negative input -4. This raises a NegativeNumberException, which you can catch in the except block and print the custom error message provided by the exception. If the input was non-negative, the else block is executed and the result is printed.
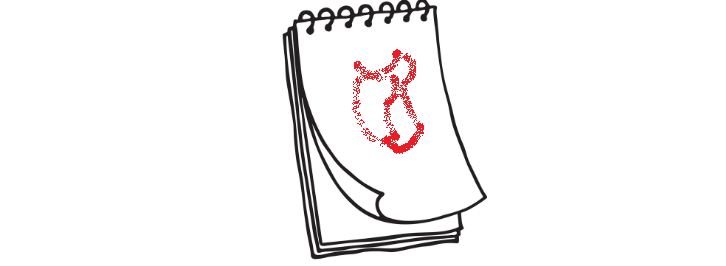
Full Source | User-defined Exceptions in Python
By defining and using custom exceptions, you can provide more specific and informative error messages for our programs, and handle errors more easily.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- How to get current date and time in Python?
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python