Python Current Date Time
In Python, you can retrieve the current date and time using the datetime module, which provides various functions and classes for working with dates and times. Here's a step-by-step explanation with examples on how to get the current date and time in Python:
Importing the datetime module
First, you need to import the datetime module in your Python script to use its functionalities.
Getting the Current Date
To obtain the current date, you can use the date.today() function from the datetime module. This function returns a date object representing the current date.
Getting the Current Time
To get the current time, you can use the datetime.datetime.now() function, which returns a datetime object representing the current date and time.
How to format Date and Time in Python?
The strftime() function is designed to insert bytes into the array, which is pointed to by the variable 's,' in accordance with the rules specified by the string denoted by 'format.' The 'format' string is a character sequence that commences and concludes in its original shift state, if applicable. It comprises a series of conversion specifications and regular characters. Each conversion specification is identified by a '%' character, optionally followed by an E or O modifier, and concludes with a termination character that governs the behavior of the conversion specification.
Syntax:Following is the syntax for strftime() method:
In the above example, %Y, %m, %d, %H, %M, and %S are format codes that represent year, month, day, hour, minute, and second, respectively. You can find a list of all available format codes in the Python documentation for the strftime() method.
The following conversion specifications are supported:
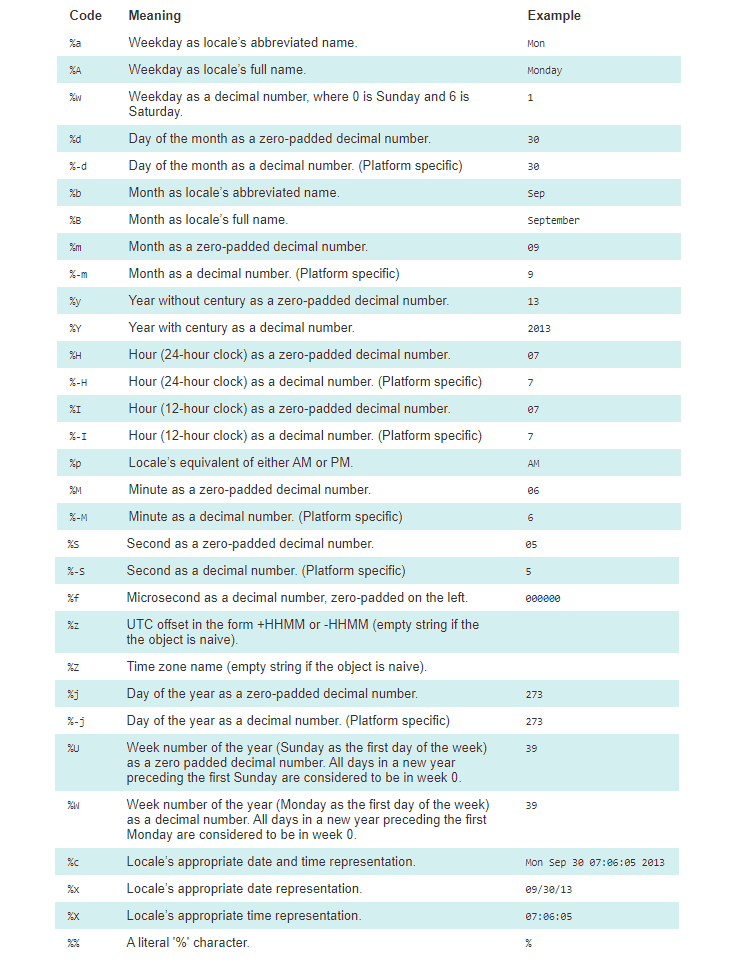
Conclusion
Using the datetime module, you can easily obtain the current date and time, and manipulate them according to your needs in Python applications.
- Python print statement "Syntax Error: invalid syntax"
- Installing Python Modules with pip
- No module named 'pip'
- How to get the length of a string in Python
- ModuleNotFoundError: No module named 'sklearn'
- ModuleNotFoundError: No module named 'cv2'
- Python was not found; run without arguments
- Attempted relative import with no known parent package
- TypeError: only integer scalar arrays can be converted to a scalar index
- A value is trying to be set on a copy of a slice from a DataFrame
- ValueError: setting an array element with a sequence
- Indentationerror: unindent does not match any outer indentation level
- Valueerror: if using all scalar values, you must pass an index
- ImportError: libGL.so.1: cannot open shared object file: No such file or directory
- Python Try Except | Exception Handling
- Custom Exceptions in Python with Examples
- Python String replace() Method
- sqrt Python | Find the Square Root in Python
- Read JSON file using Python
- Binary search in Python
- Defaultdict in Python
- Int Object is Not Iterable – Python Error
- os.path.join in Python
- TypeError: int object is not subscriptable
- Python multiline comment
- Typeerror: str object is not callable
- Python reverse List
- zip() in Python for Parallel Iteration
- strftime() in Python
- Typeerror: int object is not callable
- Python List pop() Method
- Fibonacci series in Python
- Python any() function
- Python any() Vs all()
- Python pass Statement
- Python Lowercase - String lower() Method
- Modulenotfounderror: no module named istutils.cmd
- Append to dictionary in Python : Key/Value Pair
- timeit | Measure execution time of small code
- Python Decimal to Binary
- GET and POST requests using Python
- Difference between List VS Set in Python
- How to Build Word Cloud in Python?
- Binary to Decimal in Python
- Modulenotfounderror: no module named 'apt_pkg'
- Convert List to Array Python